Bài giảng Phát triển ứng dụng web - Bài 5: Object oriented programming - Nguyễn Hữu Thể
Content
1. Class
2. Visibility
3. Properties & Methods
4. Getter & Setter
5. Create objects
6. Constructor
7. Destructor
8. Inheritance
9. Abstract class
10. Interfaces
11. Autoloading classes
12. Anonymous functions
13. Closures
14. Namespace
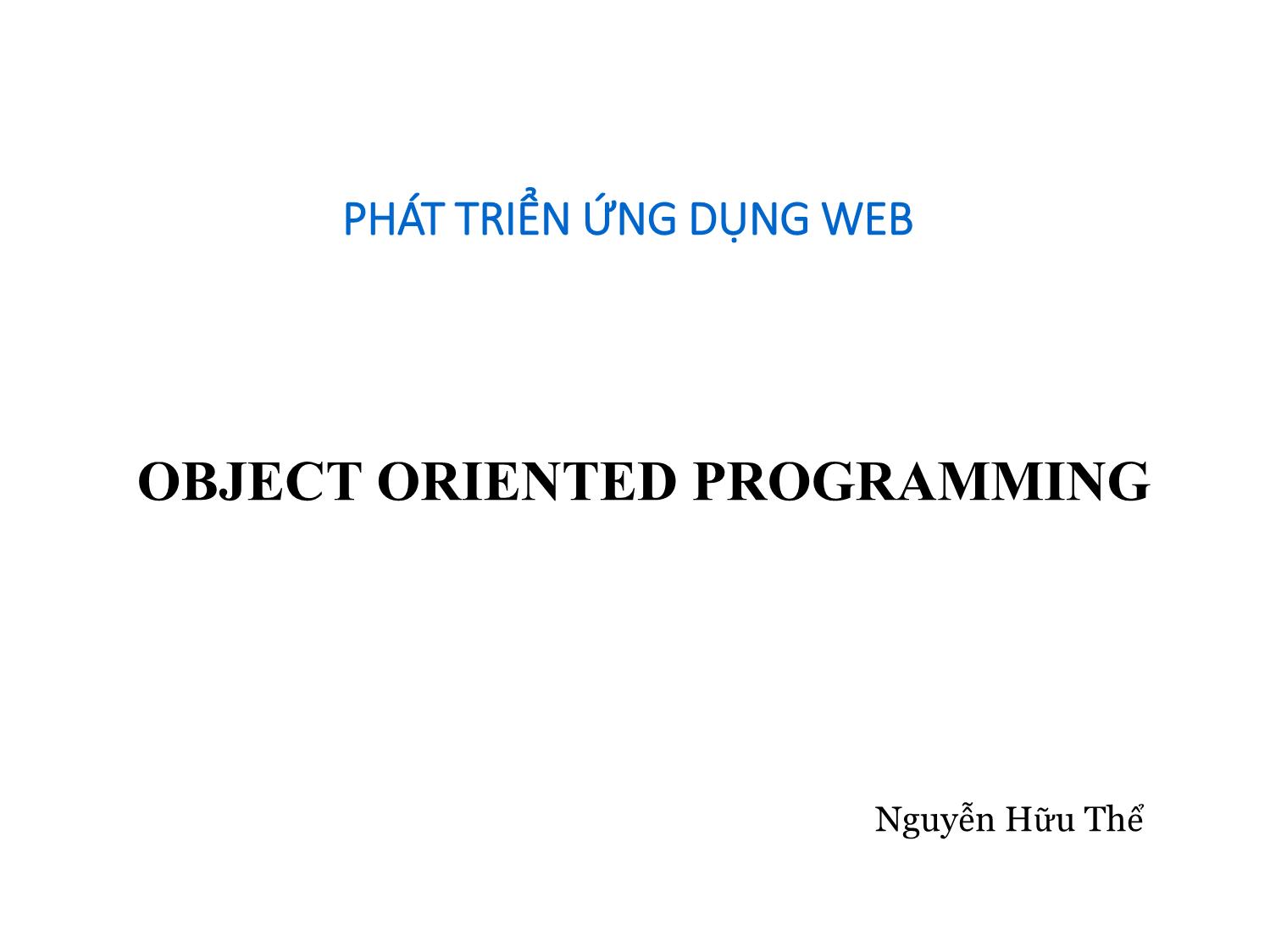
Trang 1
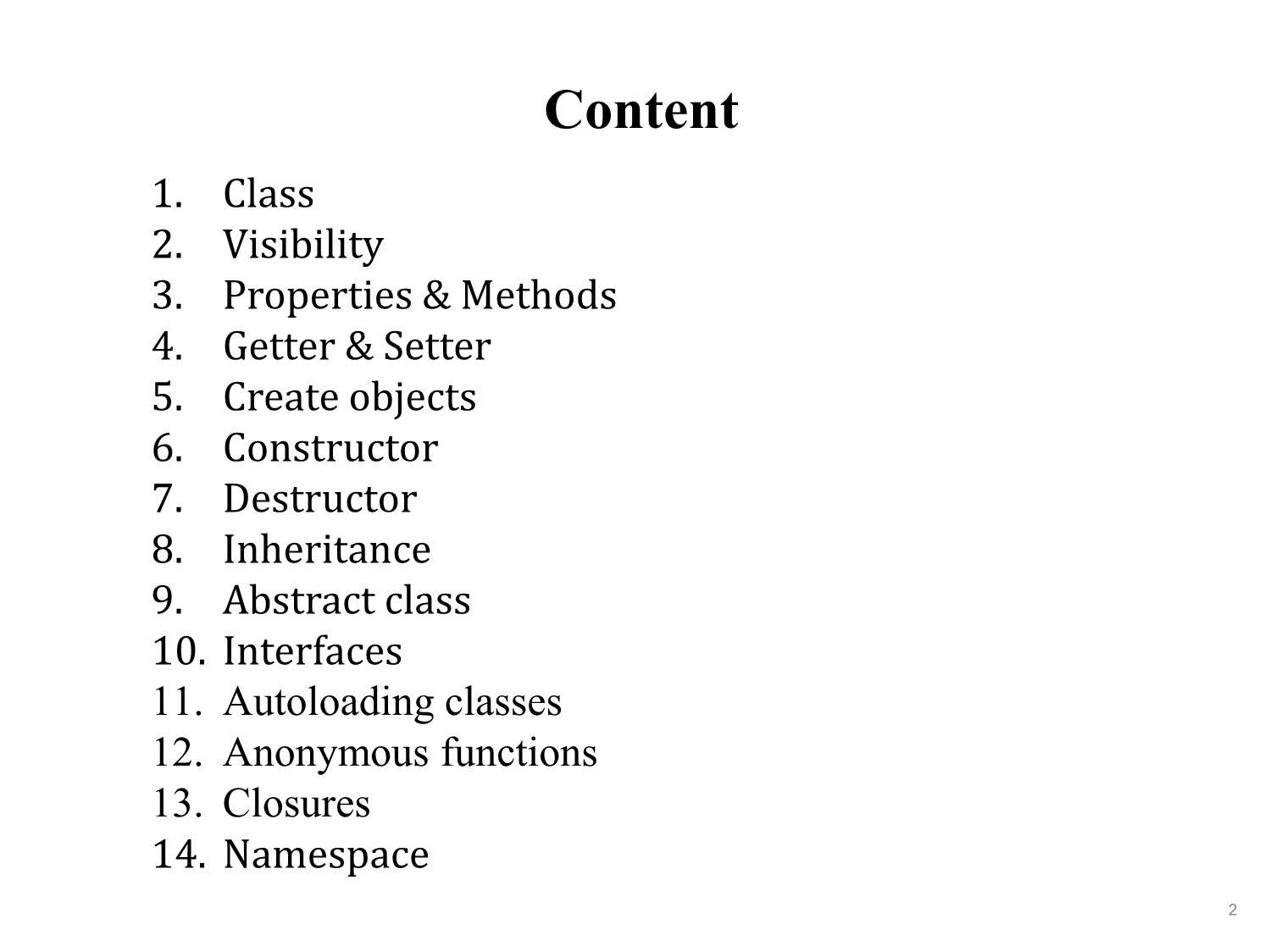
Trang 2
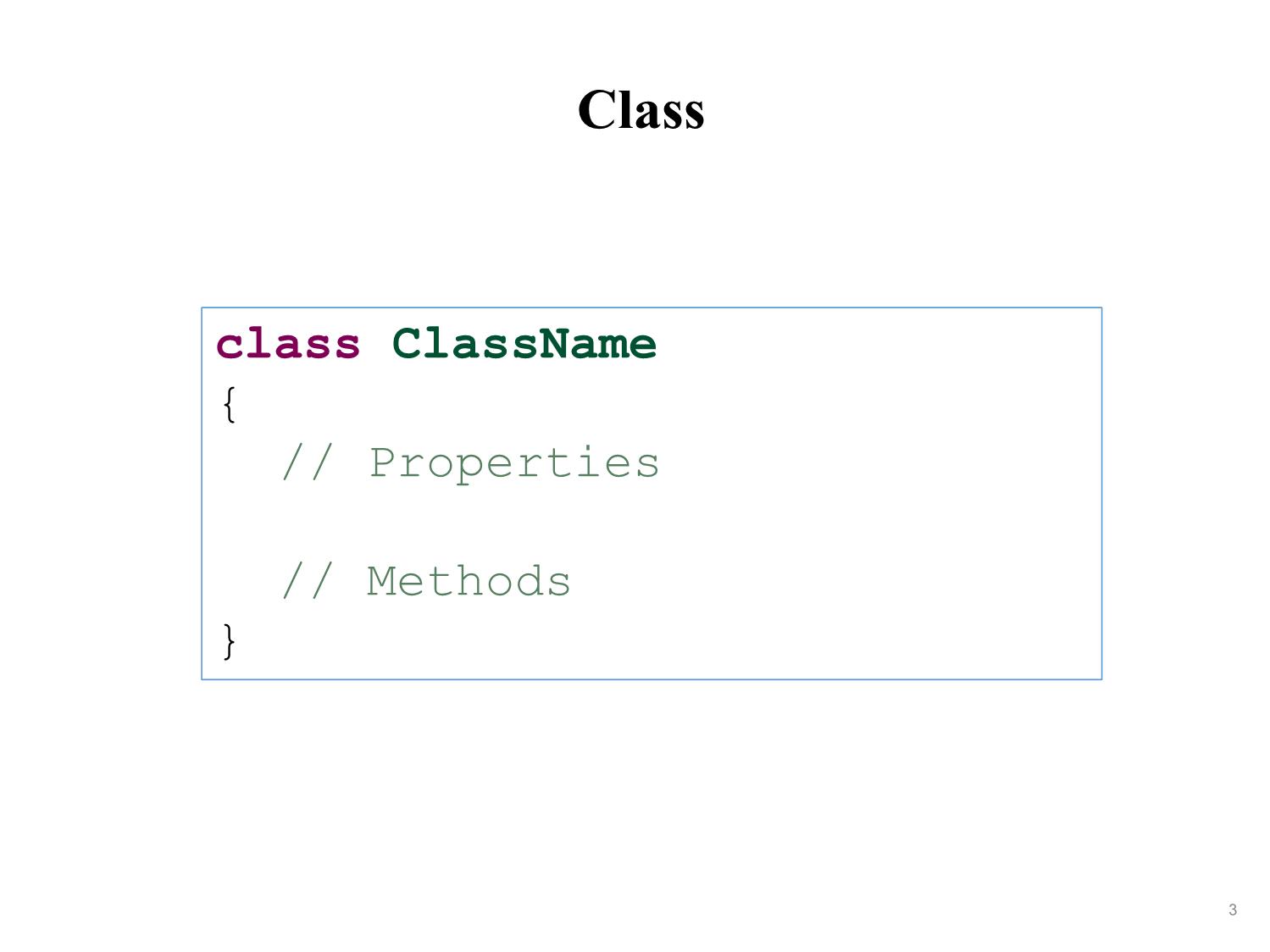
Trang 3
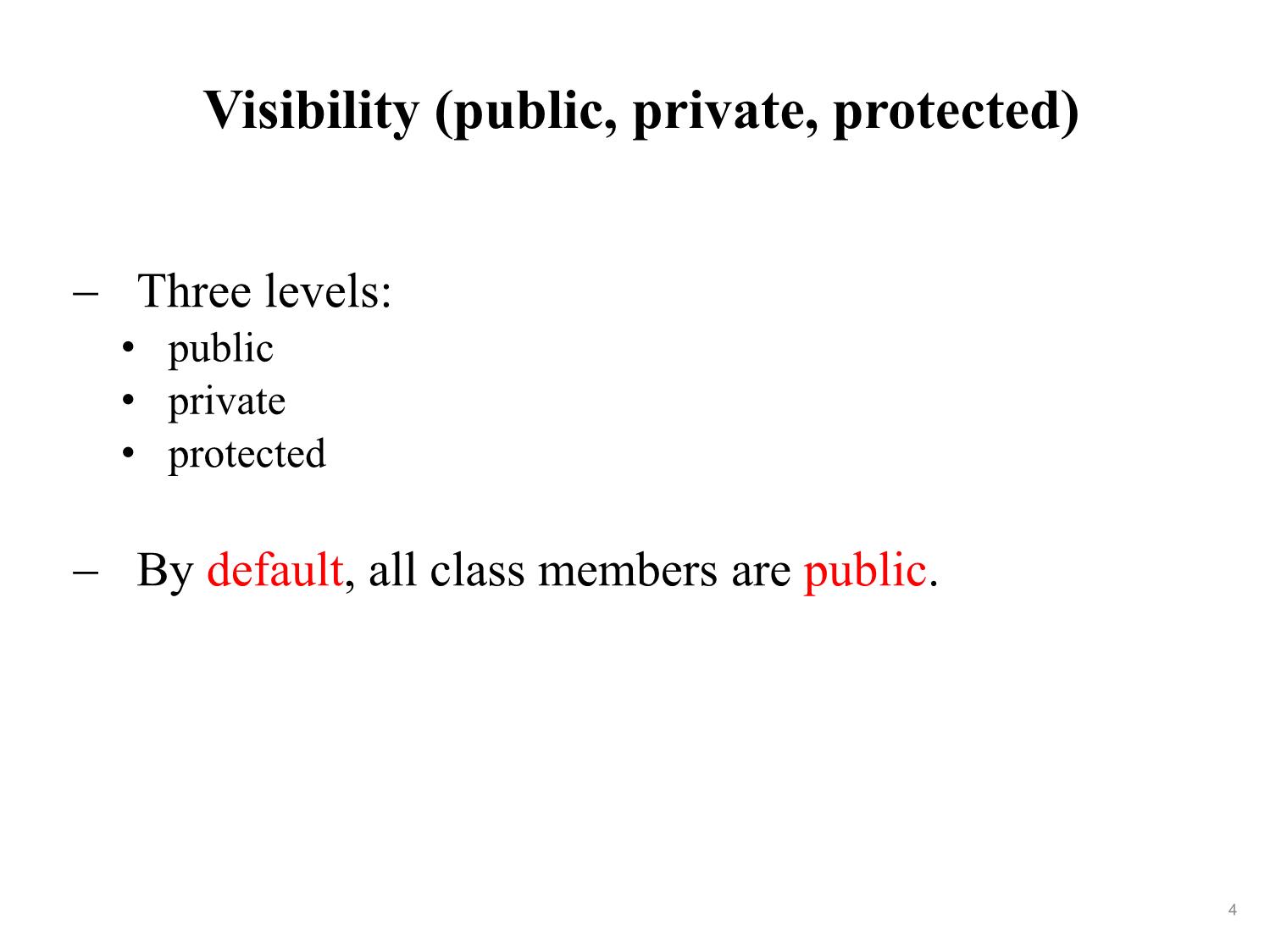
Trang 4
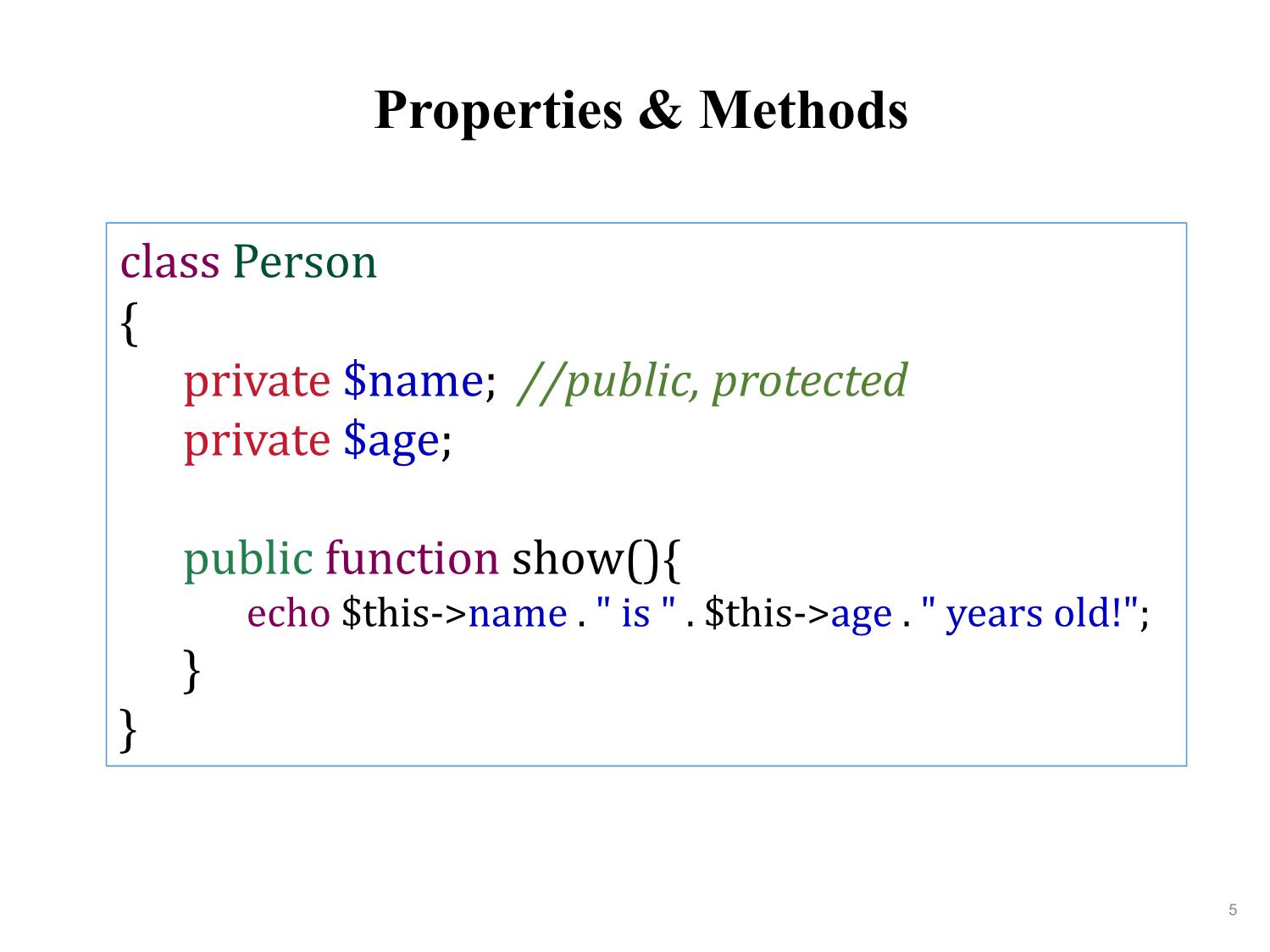
Trang 5
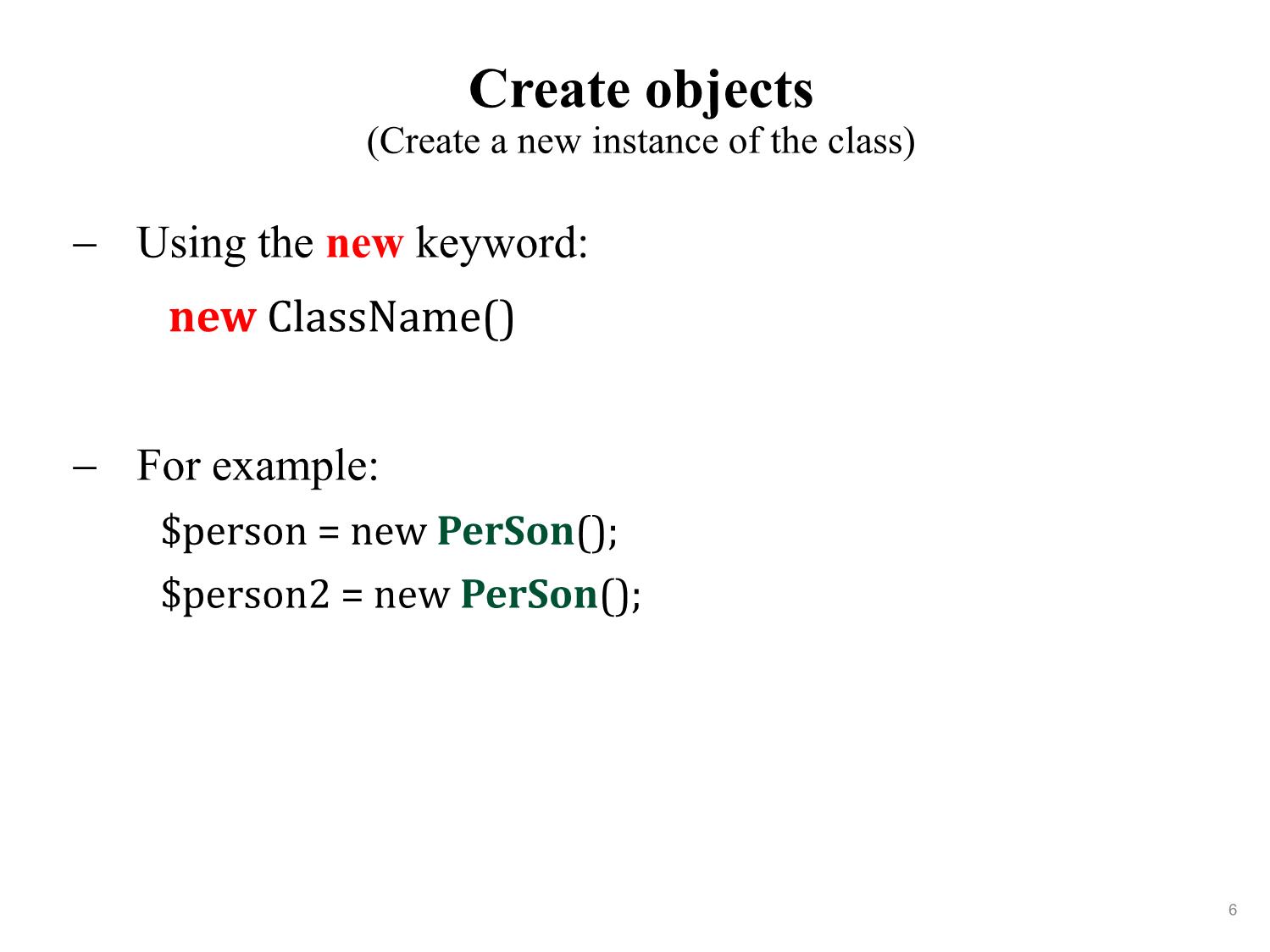
Trang 6
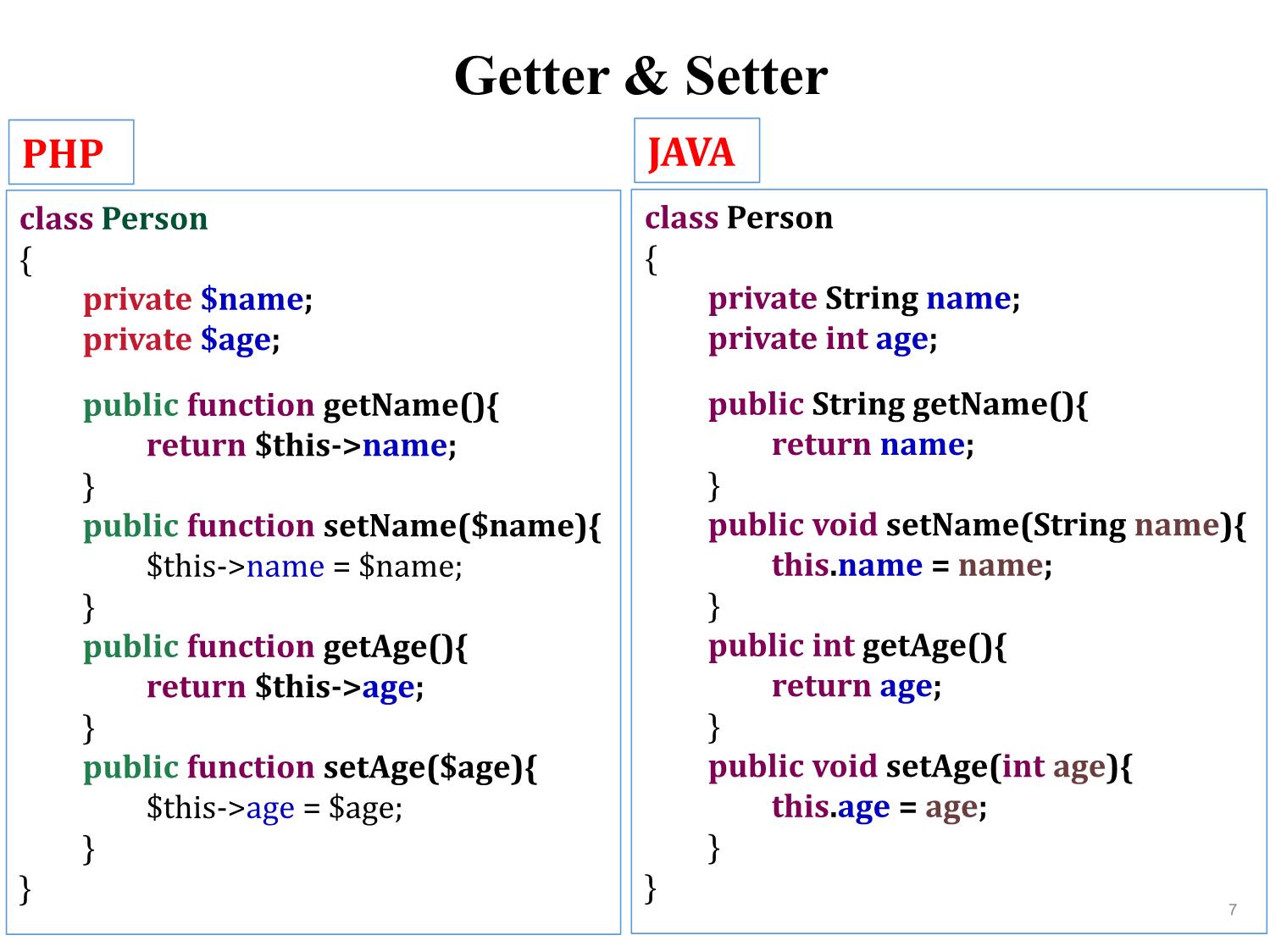
Trang 7
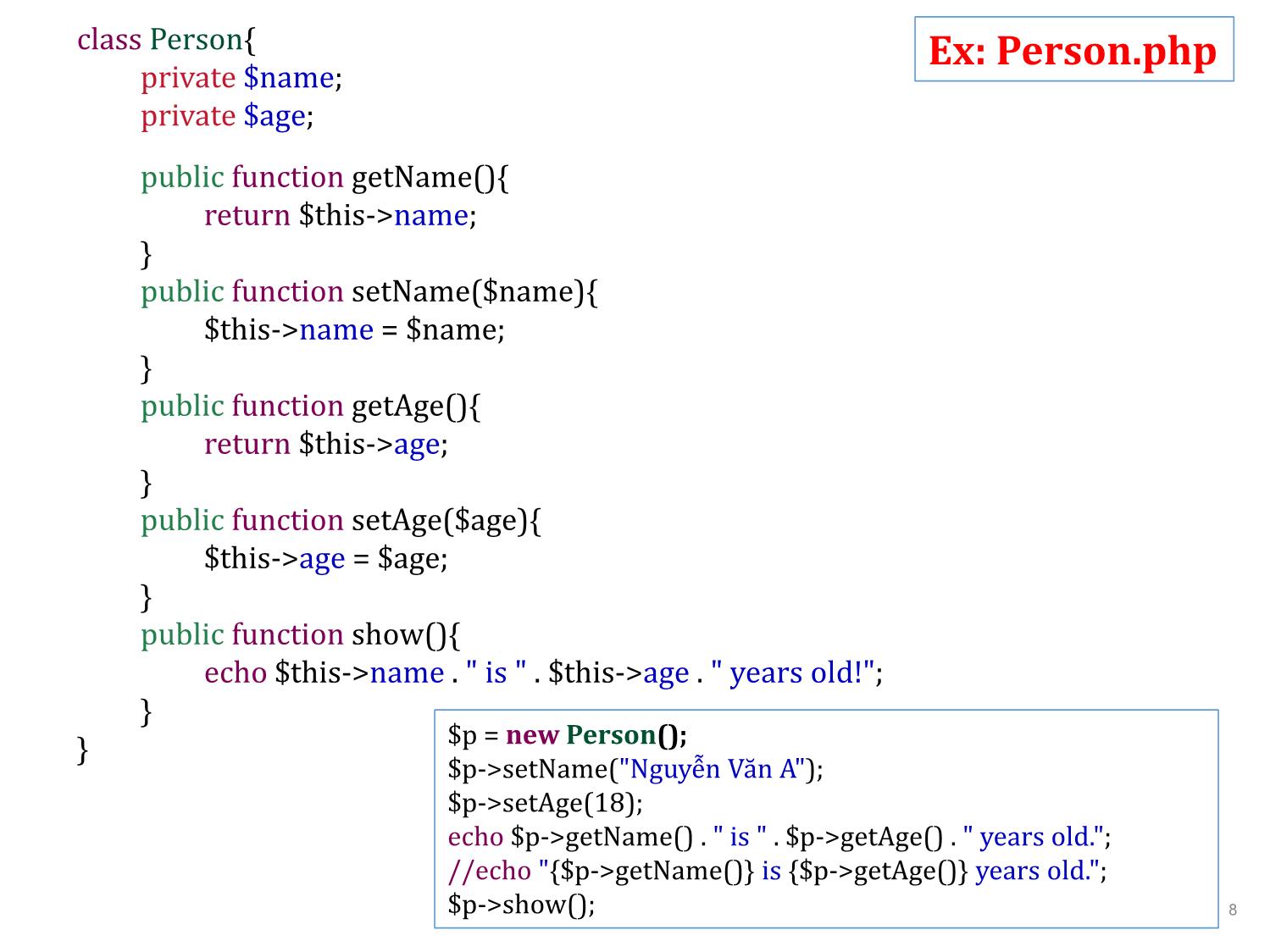
Trang 8
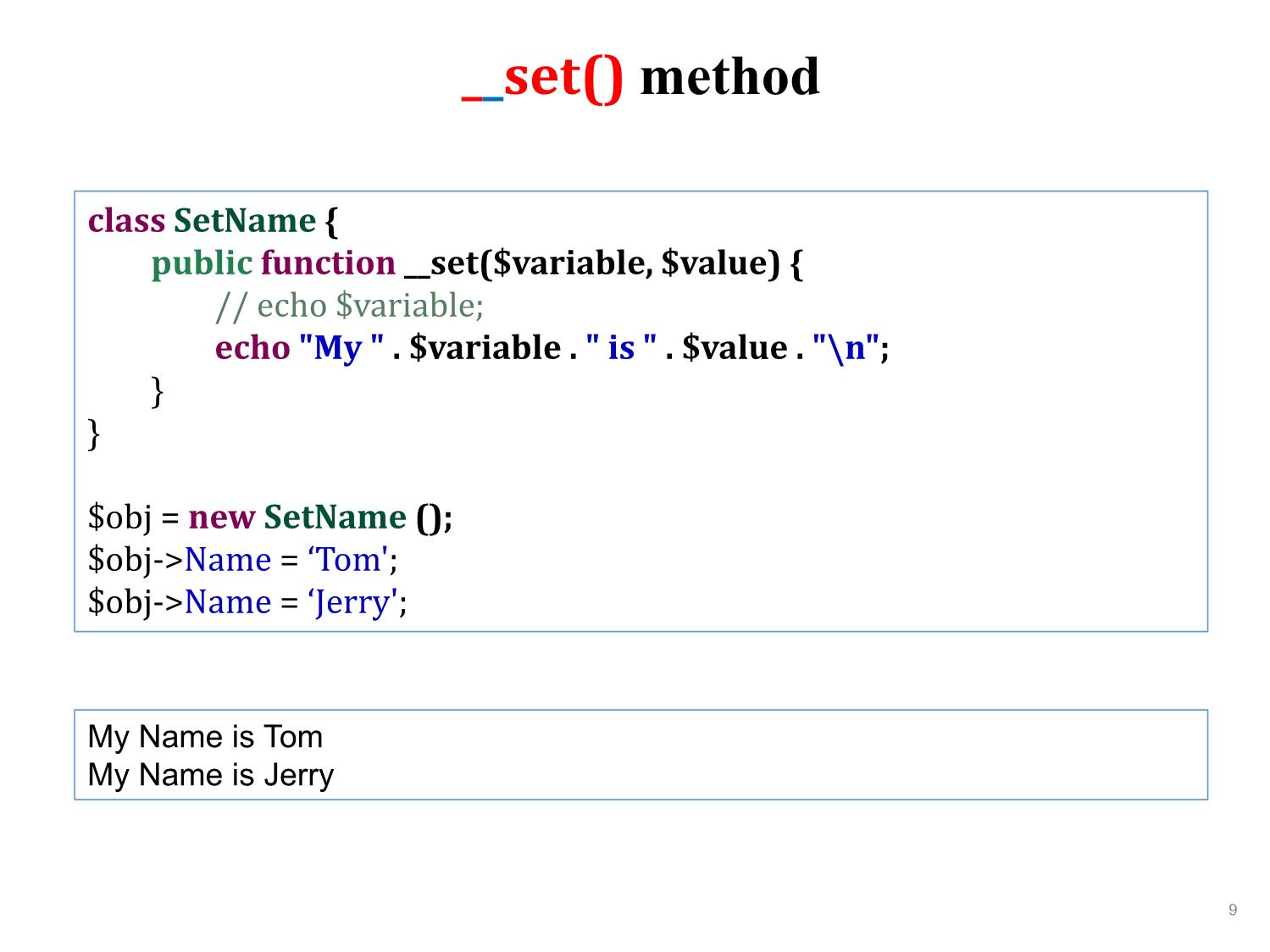
Trang 9
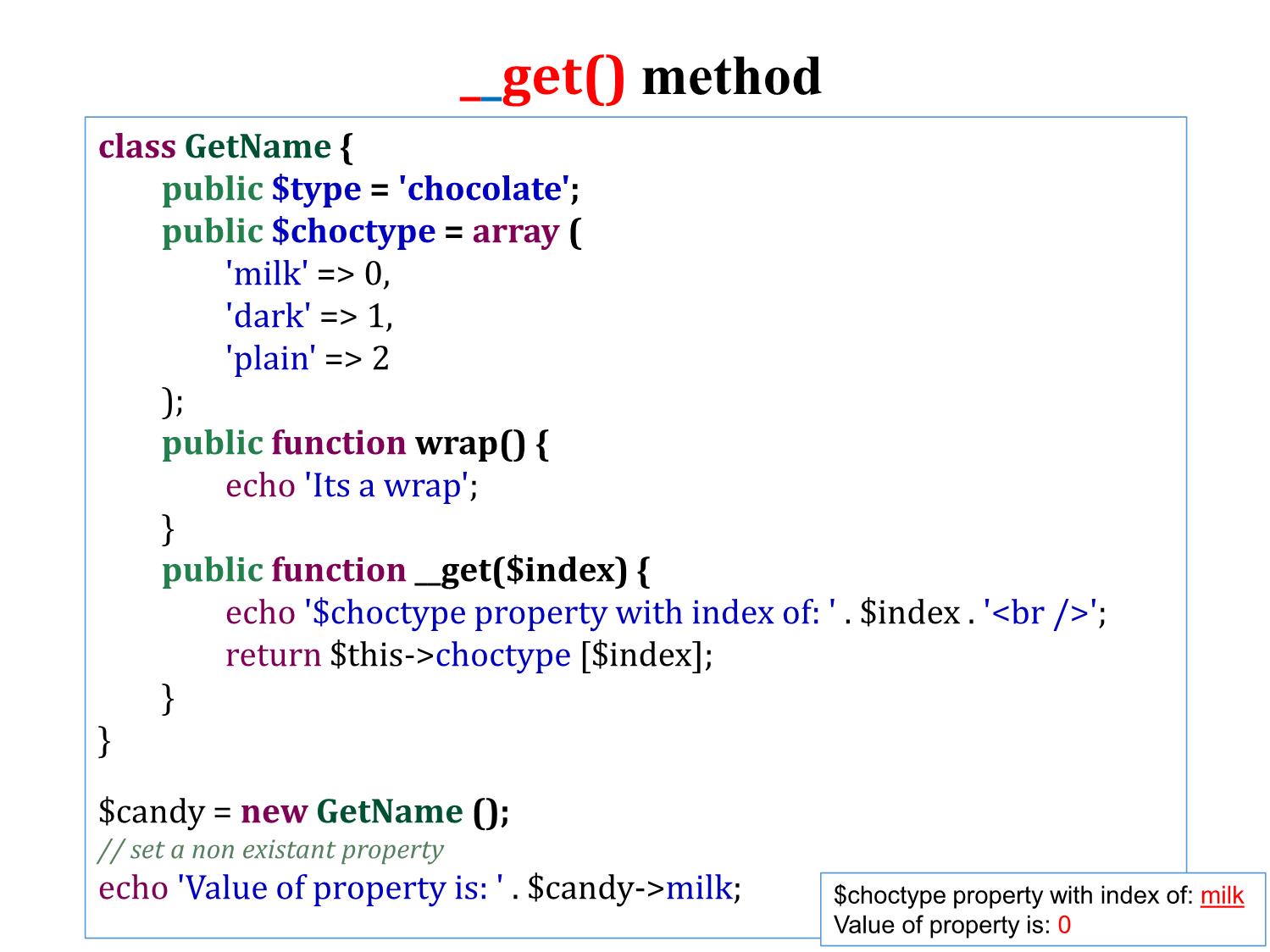
Trang 10
Tải về để xem bản đầy đủ
Bạn đang xem 10 trang mẫu của tài liệu "Bài giảng Phát triển ứng dụng web - Bài 5: Object oriented programming - Nguyễn Hữu Thể", để tải tài liệu gốc về máy hãy click vào nút Download ở trên
Tóm tắt nội dung tài liệu: Bài giảng Phát triển ứng dụng web - Bài 5: Object oriented programming - Nguyễn Hữu Thể
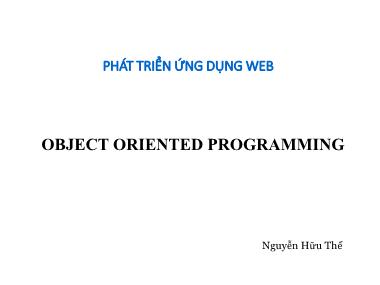
PHÁT TRIỂN ỨNG DỤNG WEB OBJECT ORIENTED PROGRAMMING Nguyễn Hữu Thể Content 1. Class 2. Visibility 3. Properties & Methods 4. Getter & Setter 5. Create objects 6. Constructor 7. Destructor 8. Inheritance 9. Abstract class 10. Interfaces 11. Autoloading classes 12. Anonymous functions 13. Closures 14. Namespace 2 Class class ClassName { // Properties // Methods } 3 Visibility (public, private, protected) − Three levels: • public • private • protected − By default, all class members are public. 4 Properties & Methods class Person { private $name; //public, protected private $age; public function show(){ echo $this->name . " is " . $this->age . " years old!"; } } 5 Create objects (Create a new instance of the class) − Using the new keyword: new ClassName() − For example: $person = new PerSon(); $person2 = new PerSon(); 6 Getter & Setter PHP JAVA class Person class Person { { private $name; private String name; private $age; private int age; public function getName(){ public String getName(){ return $this->name; return name; } } public function setName($name){ public void setName(String name){ $this->name = $name; this.name = name; } } public function getAge(){ public int getAge(){ return $this->age; return age; } } public function setAge($age){ public void setAge(int age){ $this->age = $age; this.age = age; } } } } 7 class Person{ Ex: Person.php private $name; private $age; public function getName(){ return $this->name; } public function setName($name){ $this->name = $name; } public function getAge(){ return $this->age; } public function setAge($age){ $this->age = $age; } public function show(){ echo $this->name . " is " . $this->age . " years old!"; } } $p = new Person(); $p->setName("Nguyễn Văn A"); $p->setAge(18); echo $p->getName() . " is " . $p->getAge() . " years old."; //echo "{$p->getName()} is {$p->getAge()} years old."; $p->show(); 8 __set() method class SetName { public function __set($variable, $value) { // echo $variable; echo "My " . $variable . " is " . $value . "\n"; } } $obj = new SetName (); $obj->Name = ‘Tom'; $obj->Name = ‘Jerry'; My Name is Tom My Name is Jerry 9 __get() method class GetName { public $type = 'chocolate'; public $choctype = array ( 'milk' => 0, 'dark' => 1, 'plain' => 2 ); public function wrap() { echo 'Its a wrap'; } public function __get($index) { echo '$choctype property with index of: ' . $index . ''; return $this->choctype [$index]; } } $candy = new GetName (); // set a non existant property echo 'Value of property is: ' . $candy->milk; $choctype property with index of: milk 10 Value of property is: 0 Constructor public function __construct() { //... } public function __construct($name, $age) { //... } 11 Constructor class Person{ private $name; private $age; public function __construct($name, $age) { $this->name = $name; $this->age = $age; } public function show(){ echo $this->name . " is " . $this->age . " years old!"; } } $p = new Person("Nguyễn Trần Lê", 18); $p->show(); 12 Destructor public function __destruct() { //... } − Example: public function __destruct() { echo "Bye bye!"; //... } − Using: $p = new Person("Nguyễn Trần Lê", 18); unset( $p ); // or exit: call __destruct() object $p->show(); // Object not found 13 Inheritance class ParentClass { public function myMethod() { // Method code here } } class ChildClass extends ParentClass { public function myMethod() { // For ChildClass objects, this method is called // instead of the parent class's MyMethod() } } 14 Calling a parent method from a child method parent::myMethod(); Example: parent::show(); parent::__construct(); 15 require_once 'Person.php'; class Employee extends Person{ private $salary; Inheritance public function __construct($name, $age, $salary){ parent::__construct($name, $age); // Call __construct() parent $this->salary = $salary; } public function getSalary(){ return $this-> $salary; } // Override public function show(){ parent::show(); echo " Salary: " . $this->salary; } public function display(){ echo " Name: " . $this->getName() . ""; echo " Age: " . $this->getAge() . ""; echo "Salary: " . $this->salary; } $e = new Employee("Nguyễn Lê", 20, 200); } $e->show(); Nguyễn Lê is 20 years old! Salary: 200 16 Visibility (Example 1) Property Visibility class MyClass { public $public = 'Public'; protected $protected = 'Protected'; private $private = 'Private'; function printHello() { echo $this->public; echo $this->protected; echo $this->private; } } $obj = new MyClass(); echo $obj->public; // Works echo $obj->protected; // Fatal Error echo $obj->private; // Fatal Error $obj->printHello(); // Shows Public, Protected and Private 17 Visibility (Example 2) Property Visibility class MyClass2 extends MyClass { // We can redeclare the public and protected method, but not private public $public = 'Public2'; protected $protected = 'Protected2'; function printHello() { echo $this->public; echo $this->protected; echo $this->private; } } $obj2 = new MyClass2(); echo $obj2->public; // Works echo $obj2->protected; // Fatal Error echo $obj2->private; // Undefined $obj2->printHello(); // Shows Public2, Protected2, Undefined 18 Visibility (Example 3) Method Visibility class MyClass { // Declare a public constructor public function __construct() { } // Declare a public method public function MyPublic() { } // Declare a protected method protected function MyProtected() { } // Declare a private method private function MyPrivate() { } // This is public function Foo() { $this->MyPublic(); $myclass = new MyClass; $this->MyProtected(); $myclass->MyPublic(); // Works $this->MyPrivate(); $myclass->MyProtected(); // Fatal Error } $myclass->MyPrivate(); // Fatal Error } $myclass->Foo(); // Public, Protected and Private work 19 Visibility (Example 4) Method Visibility class MyClass2 extends MyClass { // This is public function Foo2() { $this->MyPublic(); $this->MyProtected(); $this->MyPrivate(); // Fatal Error } } $myclass2 = new MyClass2; $myclass2->MyPublic(); // Works $myclass2->Foo2(); // Public and Protected work, not Private 20 Visibility (Example 5) Method Visibility class Bar class Foo extends Bar { { public function test() { public function testPublic() { $this->testPrivate(); echo "Foo::testPublic\n"; $this->testPublic(); } } private function testPrivate() { public function testPublic() { echo "Foo::testPrivate\n"; echo "Bar::testPublic\n"; } } } private function testPrivate() { $myFoo = new Foo(); echo "Bar::testPrivate\n"; $myFoo->test(); // Bar::testPrivate } // Foo::testPublic } 21 Abstract class − An abstract class is a class that cannot be instantiated on its own. abstract class Mathematics{ /*** child class must define these methods ***/ abstract protected function getMessage(); abstract protected function add($num); /** * method common to both classes **/ public function showMessage() { echo $this->getMessage(); } } 22 Abstract class require_once 'Mathematics.php'; class myMath extends Mathematics { protected function getMessage() { return "The anwser is: "; } public function add($num) { return $num + 2; } } // A new instance of myMath $myMath = new MyMath (); $myMath->showMessage (); echo $myMath->add (4); The anwser is: 6 23 Interfaces − An interface declares one or more methods. − Must be implemented by any class that implements the interface. interface MyInterface { public function aMethod(); public function anotherMethod(); } class MyClass implements MyInterface { public function aMethod() { // (code to implement the method) } public function anotherMethod() { // (code to implement the method) } } 24 interface Persistable { public function save(); public function load(); public function delete(); } require_once 'Persistable.php'; public function save() { class Member implements Persistable { echo "Saving member to private $username; database"; private $location; } private $homepage; public function load() { public function __construct( $username, echo "Loading member from $location, $homepage ) { database"; $this->username = $username; } $this->location = $location; public function delete () { $this->homepage = $homepage; echo "Deleting member from } database"; public function getUsername() { } return $this->username; } } public function getLocation() { $m = new Member("Aha", "VN", "No page"); return $this->location; echo $m->getUsername() . ""; } $m->load(); public function getHomepage() { //... return $this->homepage; Aha } Loading member from database class Topic implements Persistable { private $subject; private $author; private $createdTime; public function __construct( $subject, $author ) { $this->subject = $subject; $this->author = $author; $this->createdTime = time(); } public function showHeader() { $createdTimeString = date( 'l jS M Y h:i:s A', $this->createdTime ); $authorName = $this->author->getUsername(); echo "$this->subject (created on $createdTimeString by $authorName)"; } public function save() { echo "Saving topic to database"; } public function load() { echo "Loading topic from database"; } public function delete () { echo "Deleting topic from database"; } } Test.php require_once 'Member.php'; require_once 'Topic.php'; $aMember = new Member ( "TUI", "Viet Nam", "" ); echo $aMember->getUsername () . " lives in " . $aMember->getLocation () . ""; $aMember->save (); $aTopic = new Topic ( "PHP is Great", $aMember ); $aTopic->showHeader (); $aTopic->save (); TUI lives in Viet Nam Saving member to database PHP is Great (created on Saturday 17th Dec 2016 03:00:07 AM by TUI) Saving topic to database Autoloading classes − Create a function called __autoload() near the start of your PHP application. − PHP automatically calls your __autoload() function. function __autoload($className) { require_once ("$className.php"); echo "Loaded $className.php"; } $member = new Member("TUI", "Viet Nam", "No page"); echo "Created object: "; print_r ( $member ); Loaded Member.php Created object: Member Object ( [username:Member:private] => TUI [location:Member:private] => Viet Nam [homepage:Member:private] => No page ) 28 Anonymous functions − Anonymous functions have no name. // Assign an anonymous function to a variable $makeGreeting = function ($name, $timeOfDay) { return ('Good ' . $timeOfDay . ', ' . $name); }; // Call the anonymous function echo $makeGreeting ( 'Tom', 'morning' ) . ''; echo $makeGreeting ( 'Jerry', 'afternoon' ) . ''; Good morning, Tom Good afternoon, Jerry 29 Closures − A closure is an anonymous function that can access variables imported from the outside scope without using any global variables. function myClosure($num) { return function ($x) use ($num) { return $num * $x; }; } $closure = myClosure ( 10 ); echo $closure ( 2 ); echo $closure ( 3 ); 20 30 30 Namespaces − Namespaced code is defined using a singlenamespace keyword at the top of your PHP file. − It must be the first command (with the exception ofdeclare) and no non-PHP code, HTML, or white-space // define this code in the 'MyProject' namespace namespace MyProject; // ... code ... 31 Cat.php Animal.php namespace foo; namespace other\animate; class Cat { class Animal { static function says() { static function breathes() { echo 'Meoow'; echo 'Air'; } } } } Dog.php Test.php namespace bar; namespace test; class Dog { include 'Cat.php'; static function says() { include 'Dog.php'; echo 'Ruff'; include 'Animal.php'; } use foo as cat; } use bar as dog; //use other\animate; use other\animate as animal; Result: echo cat\Cat::says (), ""; Meoow echo dog\Dog::says (), ""; Ruff echo animal\Animal::breathes (); Air //echo \other\animate\Animal::breathes ();
File đính kèm:
bai_giang_phat_trien_ung_dung_web_bai_5_object_oriented_prog.pdf