Bài giảng Lập trình trên Windows với Microsoft.NET - Phần 4
public class Point
{
public int m_x;
public int m_y;
public Point (){ }
public Point(int xx,int yy)
{
m_x = xx ;
m_y = yy;
}
public static Point operator + (Point p1,Point p2) {
Point result = new Point();
result.m_x = p1.m_x + p2.m_y;
result.m_y = p1.m_x + p2.m_y;
return result;
}
}
static void Main(string[] args)
{
Point objP1 = new Point(1,1);
Point objP2 = new Point(2,2);
Point objResult = new Point();
objResult = objP1 + objP2;
Console.WriteLine("The result is m_x = {0} and m_y = {1}", objResult.m_x , objResult.m_y);
}
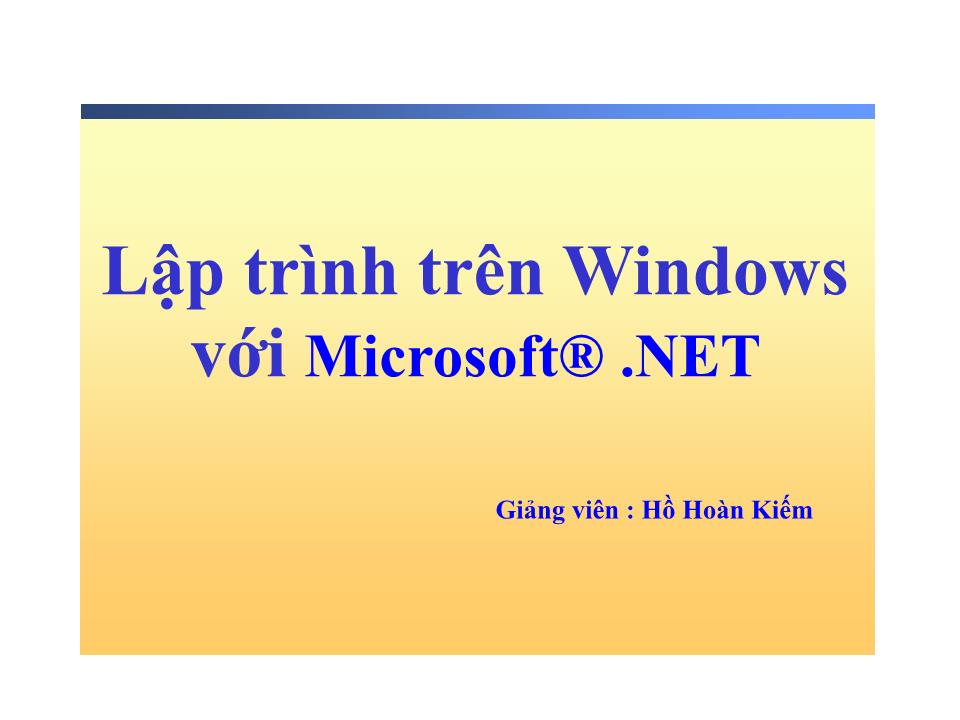
Trang 1
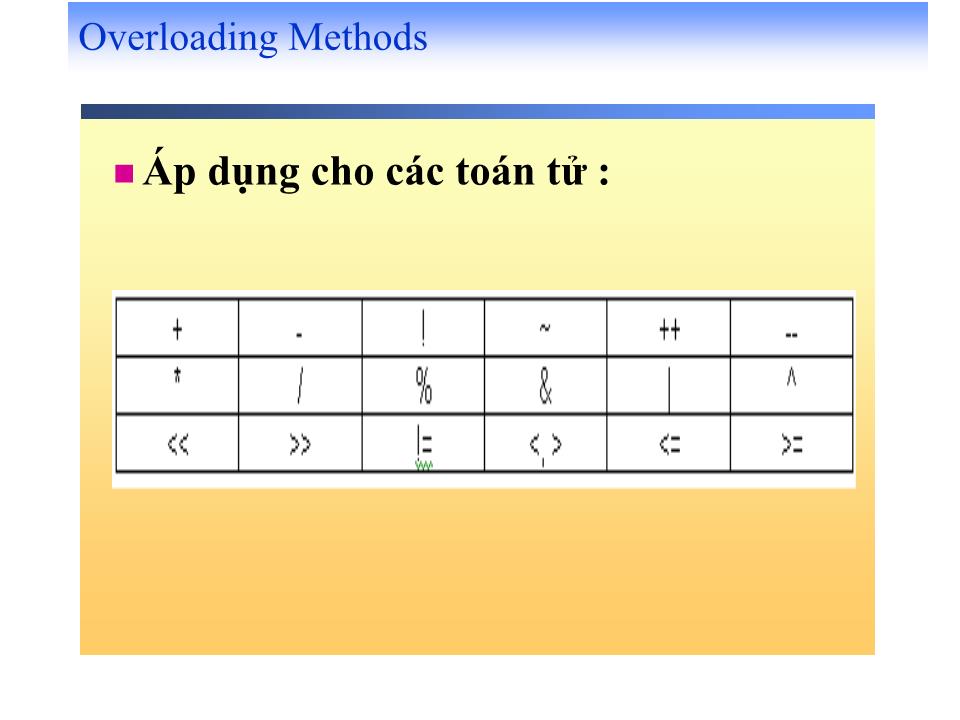
Trang 2
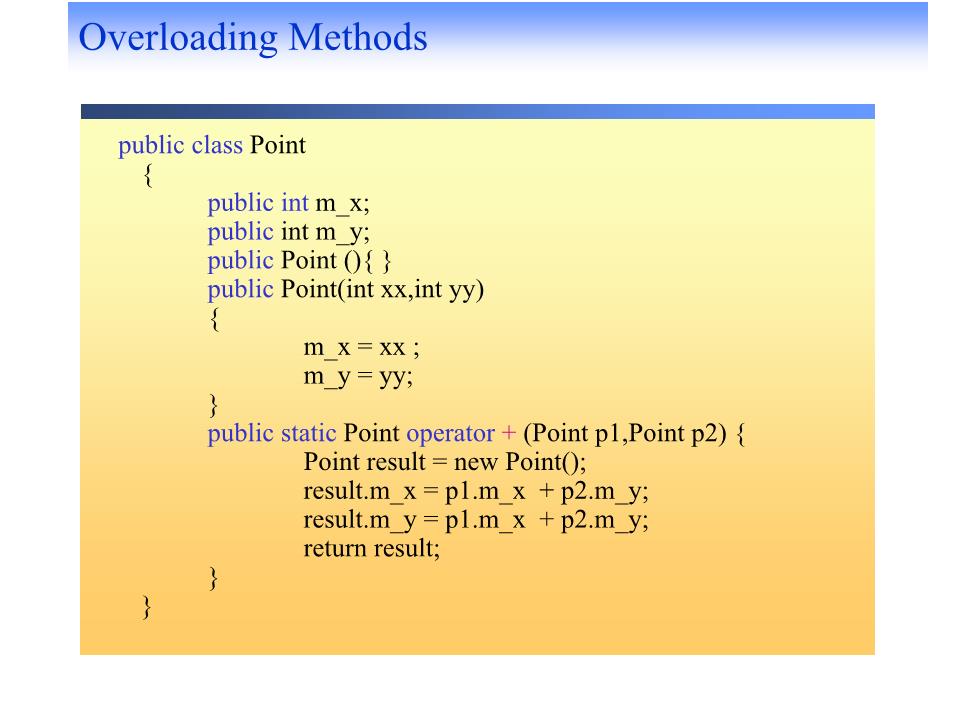
Trang 3
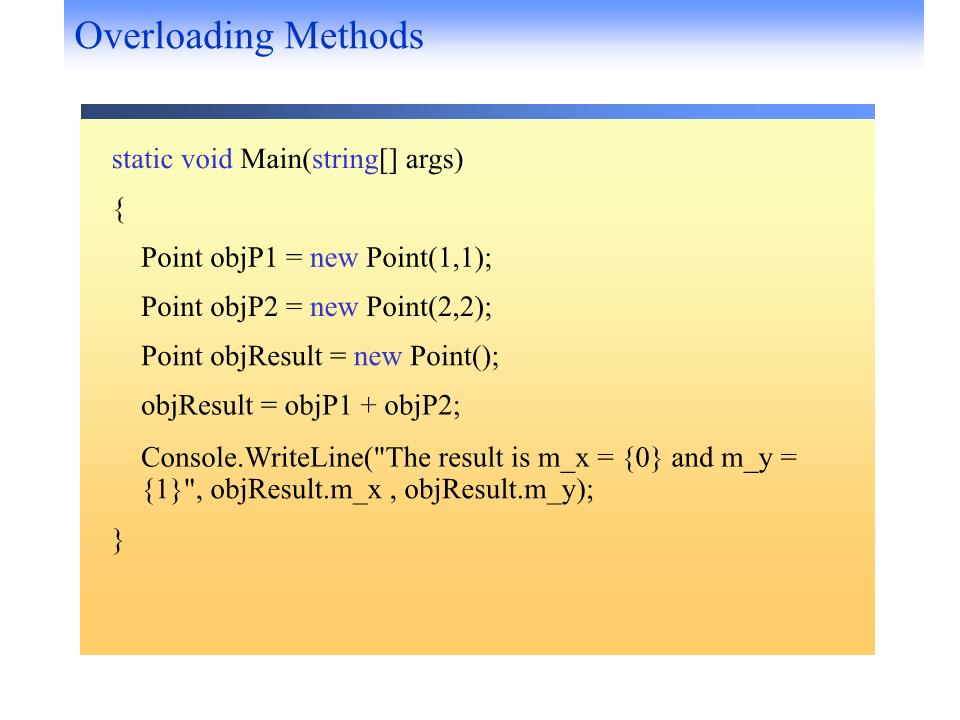
Trang 4
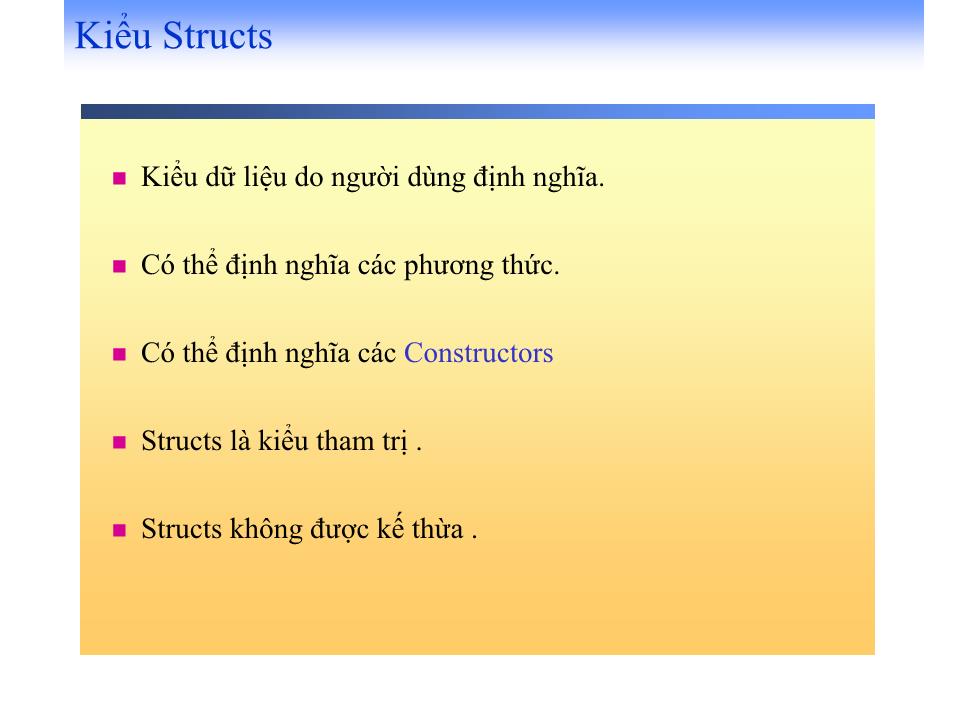
Trang 5
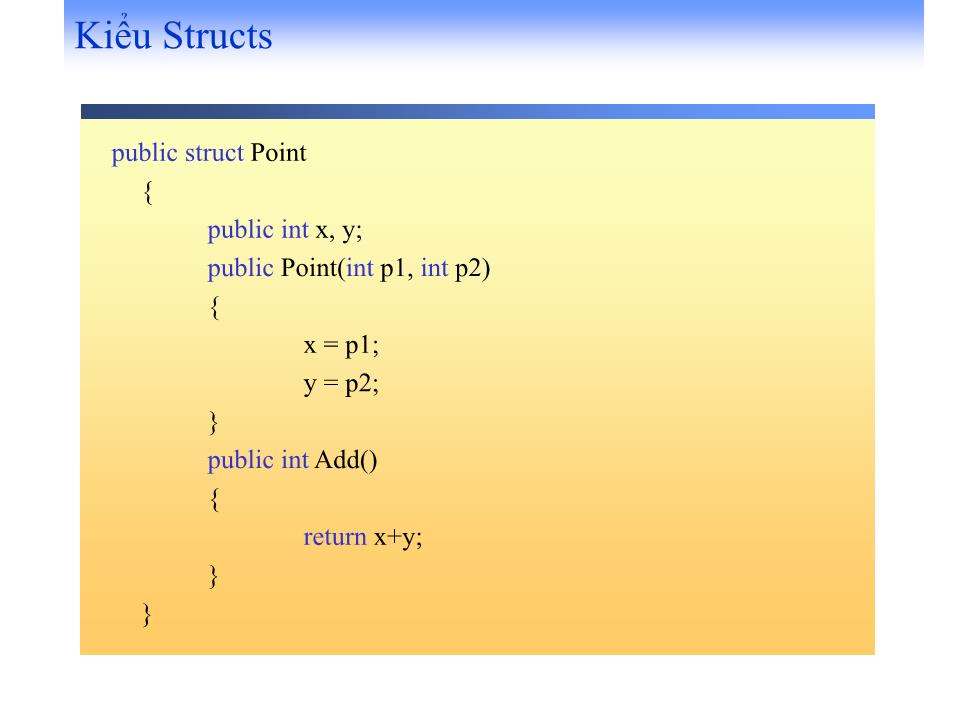
Trang 6
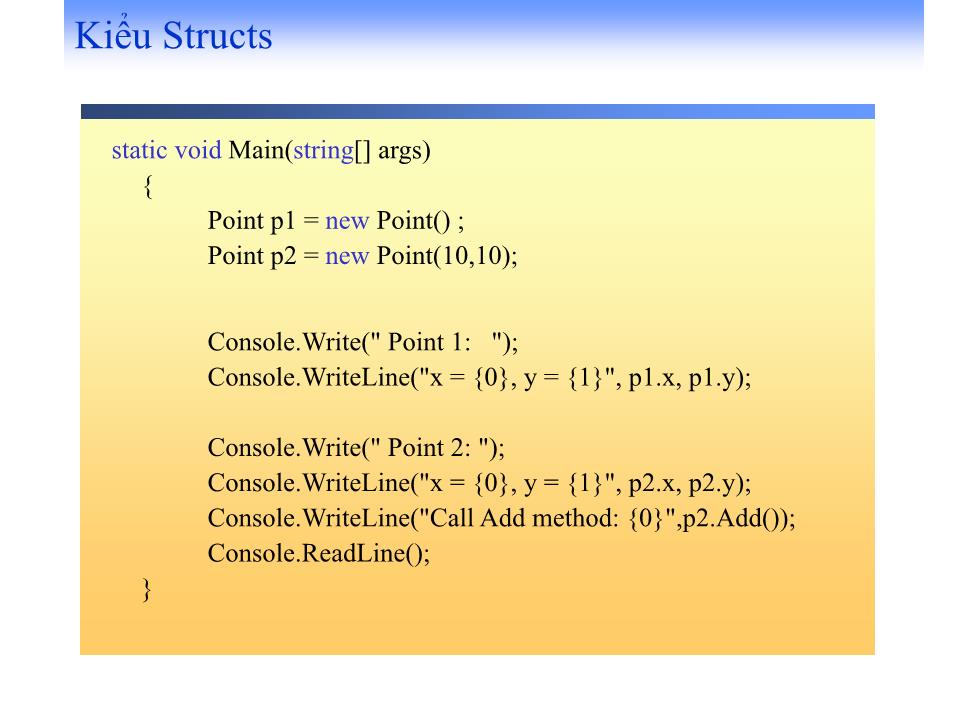
Trang 7
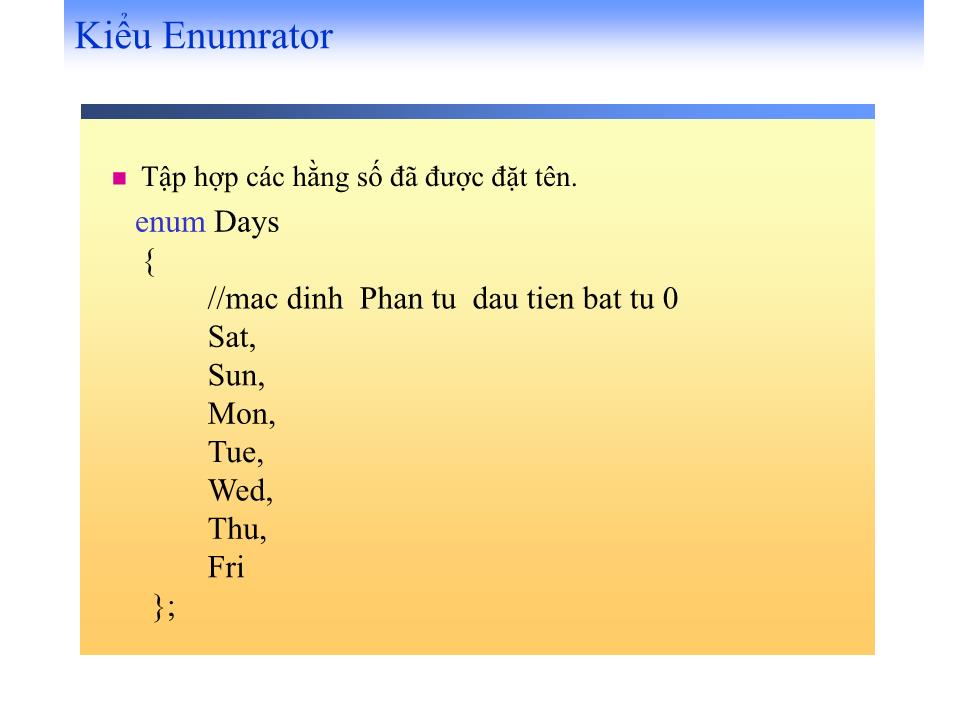
Trang 8
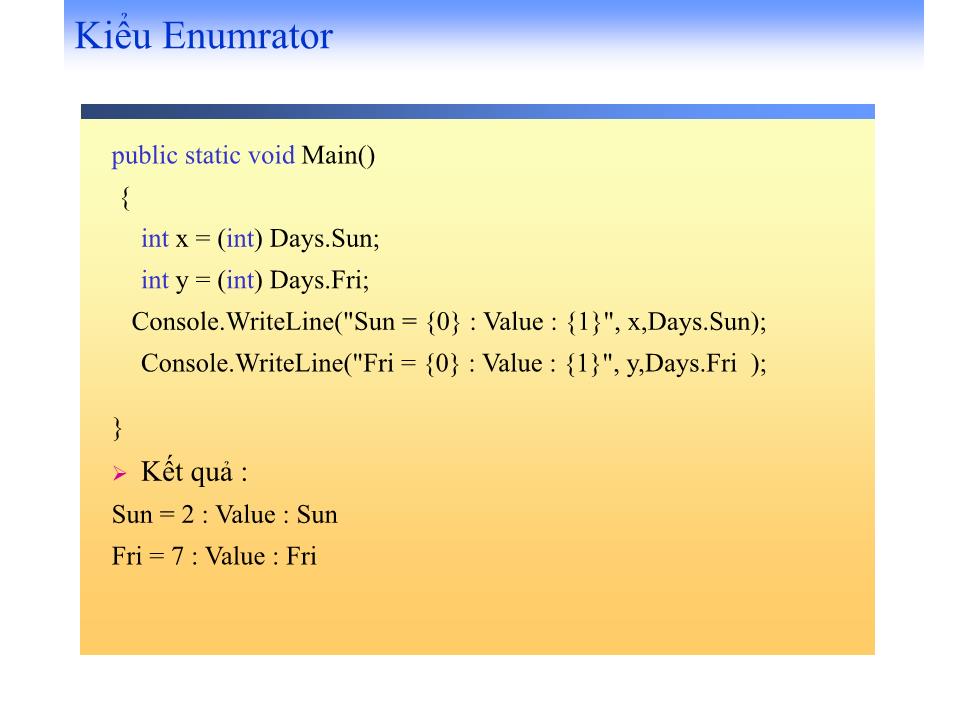
Trang 9
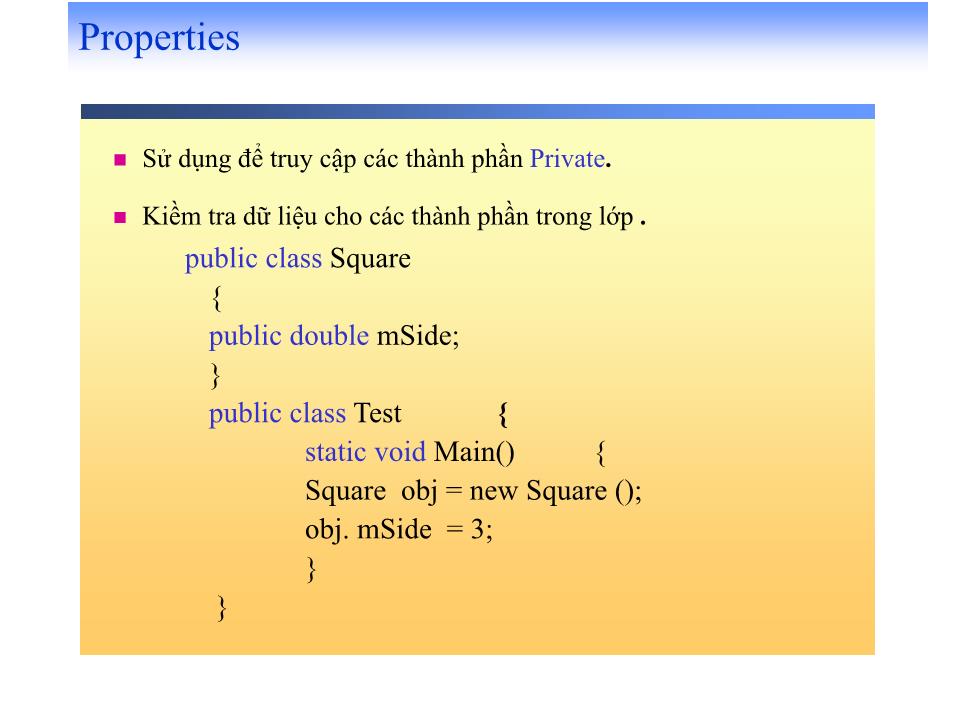
Trang 10
Tải về để xem bản đầy đủ
Bạn đang xem 10 trang mẫu của tài liệu "Bài giảng Lập trình trên Windows với Microsoft.NET - Phần 4", để tải tài liệu gốc về máy hãy click vào nút Download ở trên
Tóm tắt nội dung tài liệu: Bài giảng Lập trình trên Windows với Microsoft.NET - Phần 4
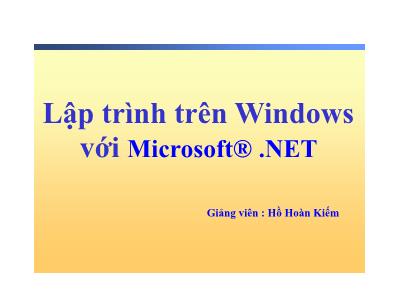
Lập trình trên Windows với Microsoft® .NET Giảng viên : Hồ Hoàn Kiếm Overloading Methods Áp dụng cho các toán tử : Overloading Methods public class Point { public int m_x; public int m_y; public Point (){ } public Point(int xx,int yy) { m_x = xx ; m_y = yy; } public static Point operator + (Point p1,Point p2) { Point result = new Point(); result.m_x = p1.m_x + p2.m_y; result.m_y = p1.m_x + p2.m_y; return result; } } Overloading Methods static void Main( string [] args) { Point objP1 = new Point(1,1); Point objP2 = new Point(2,2); Point objResult = new Point(); objResult = objP1 + objP2; Console.WriteLine("The result is m_x = {0} and m_y = {1}", objResult.m_x , objResult.m_y); } Kiểu Structs Kiểu dữ liệu do người dùng định nghĩa. Có thể định nghĩa các phương thức. Có thể định nghĩa các Constructors Structs là kiểu tham trị . Structs không được kế thừa . Kiểu Structs public struct Point { public int x, y; public Point( int p1, int p2) { x = p1; y = p2; } public int Add() { return x+y; } } Kiểu Structs static void Main( string [] args) { Point p1 = new Point() ; Point p2 = new Point(10,10); Console.Write(" Point 1: "); Console.WriteLine("x = {0}, y = {1}", p1.x, p1.y); Console.Write(" Point 2: "); Console.WriteLine("x = {0}, y = {1}", p2.x, p2.y); Console.WriteLine("Call Add method: {0}",p2.Add()); Console.ReadLine(); } Kiểu Enumrator Tập hợp các hằng số đã được đặt tên. enum Days { //mac dinh Phan tu dau tien bat tu 0 Sat, Sun, Mon, Tue, Wed, Thu, Fri }; Kiểu Enumrator public static void Main() { int x = ( int ) Days.Sun; int y = ( int ) Days.Fri; Console.WriteLine("Sun = {0} : Value : {1}", x,Days.Sun); Console.WriteLine("Fri = {0} : Value : {1}", y,Days.Fri ); } Kết quả : Sun = 2 : Value : Sun Fri = 7 : Value : Fri Properties Sử dụng để truy cập các thành phần Private . Kiềm tra dữ liệu cho các thành phần trong lớp . public class Square { public double mSide; } public class Test { static void Main() { Square obj = new Square (); obj. mSide = 3; } } Properties public class Square { //Khi báo các thành phần private double mSide; // Khai báo property public double Side { get { }; set { }; } // Khai báo các phương thức} Properties Property giống như field , nhưng việc truy cập đươc thực hiện qua thao tác get và set . public int Side { get { return mSide; } set { if (mSide < 0) return ; mSide = value ; } } Properties namespace SampleProperty { public class Rectangle { private int m_Length ; private int m_Width ; private int m_Area ; public Rectangle() { m_Length = 3; // Length = 3; m_Width = 2; } Properties public int Length { get { return m_Length; } set { if (m_Length < 0 ) return ; m_length = value ; } } Properties public int Width { ge t { return m_Width; } set { // Đọan mã kiểm tra giá trị .. m_Width = value ; } } Properties public int Area { get { return m_Area; } } public void CalArea() { m_Area = m_Length*m_Width; } Properties public class Test { public static void Main() { Rectangle objRectangle = new Rectangle(); objRectangle. Length = 3; objRectangle. Width = 4; objRectangle.CalArea(); Console.WriteLine("{0}", objRectangle. Area ); } } Indexer Khi thành phần của lớp là các kiểu tập hợp. Sử dụng với : new, virtual, sealed, override, abstract, extern . Indexer giống như Property , đuợc truy cập thông qua get và set Khai báo sử dụng từ khóa this. Khi sử dụng Indexer ta sử dụng dấu [] cho get và set . Indexer class IndexerClass { private int [] myArray = new int [100]; public int this [ int index] { get { If (index = 100) return 0; else return myArray[index]; } set { if (!(index = 100)) myArray[index] = value ; } } } Indexer public class Test { public static void Main() { IndexerClass b = new IndexerClass(); b[3] = 256; b[5] = 1024; for ( int i=0; i<=10; i++) { Console.WriteLine("Element # {0} = {1}", i, b[i]); } } }
File đính kèm:
bai_giang_lap_trinh_tren_windows_voi_microsoft_net_phan_4.ppt