Bài giảng Introduction to Computer Programming (C language) - Chapter 9: File Processing - Võ Thị Ngọc Châu
Content
Introduction
Declare files
Open and close files
Store and retrieve data from files
Use macros
Summary
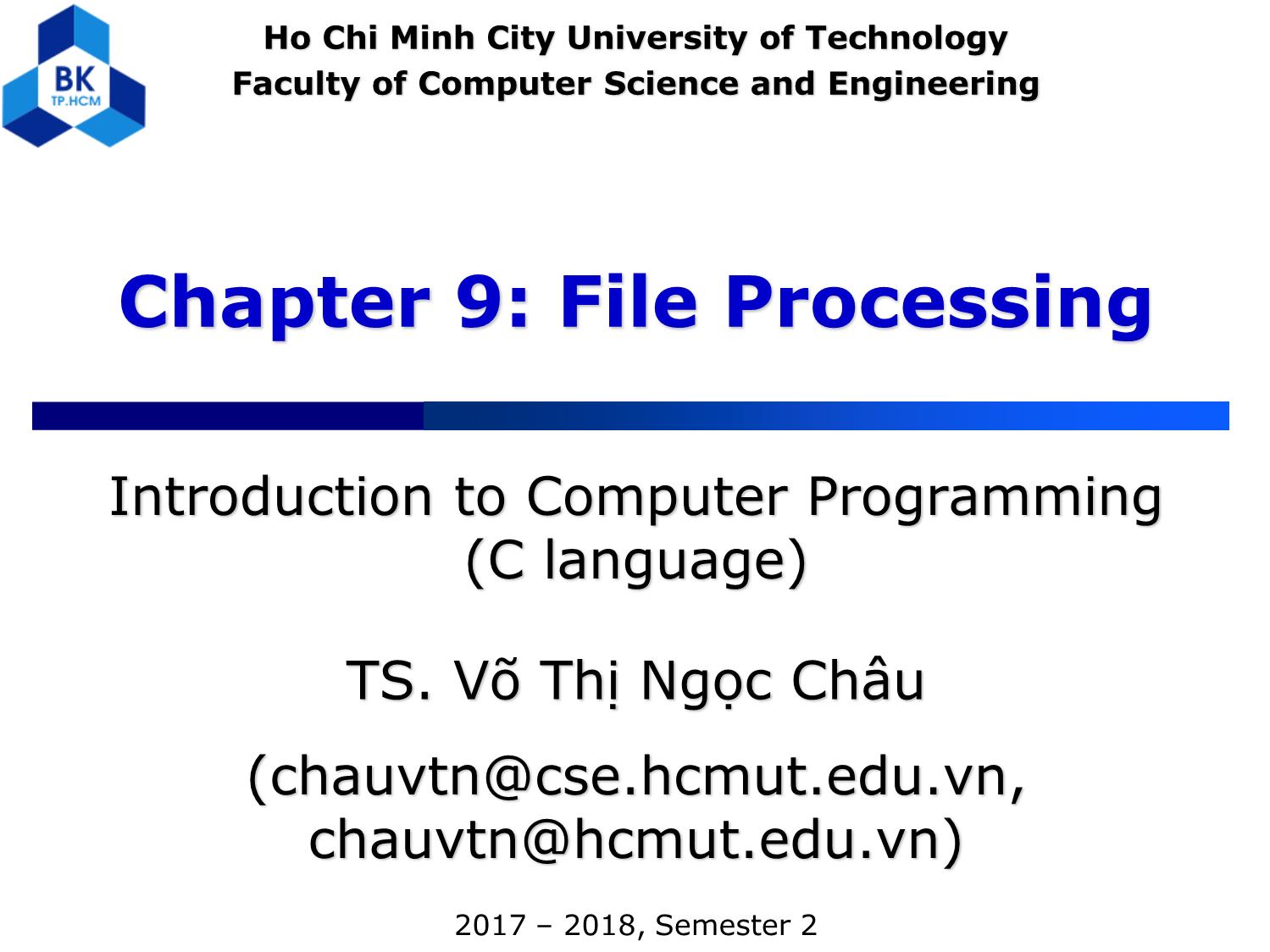
Trang 1
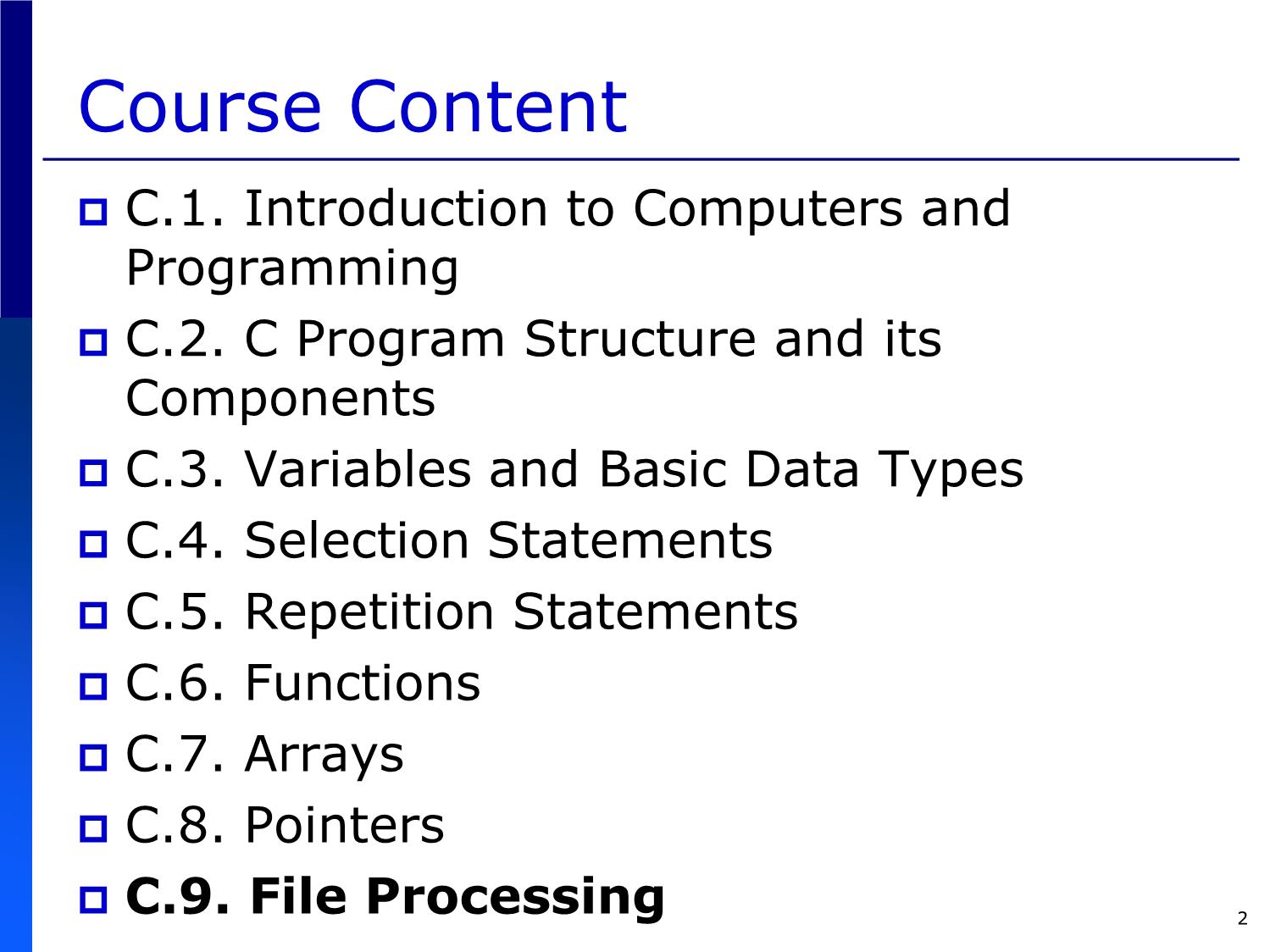
Trang 2
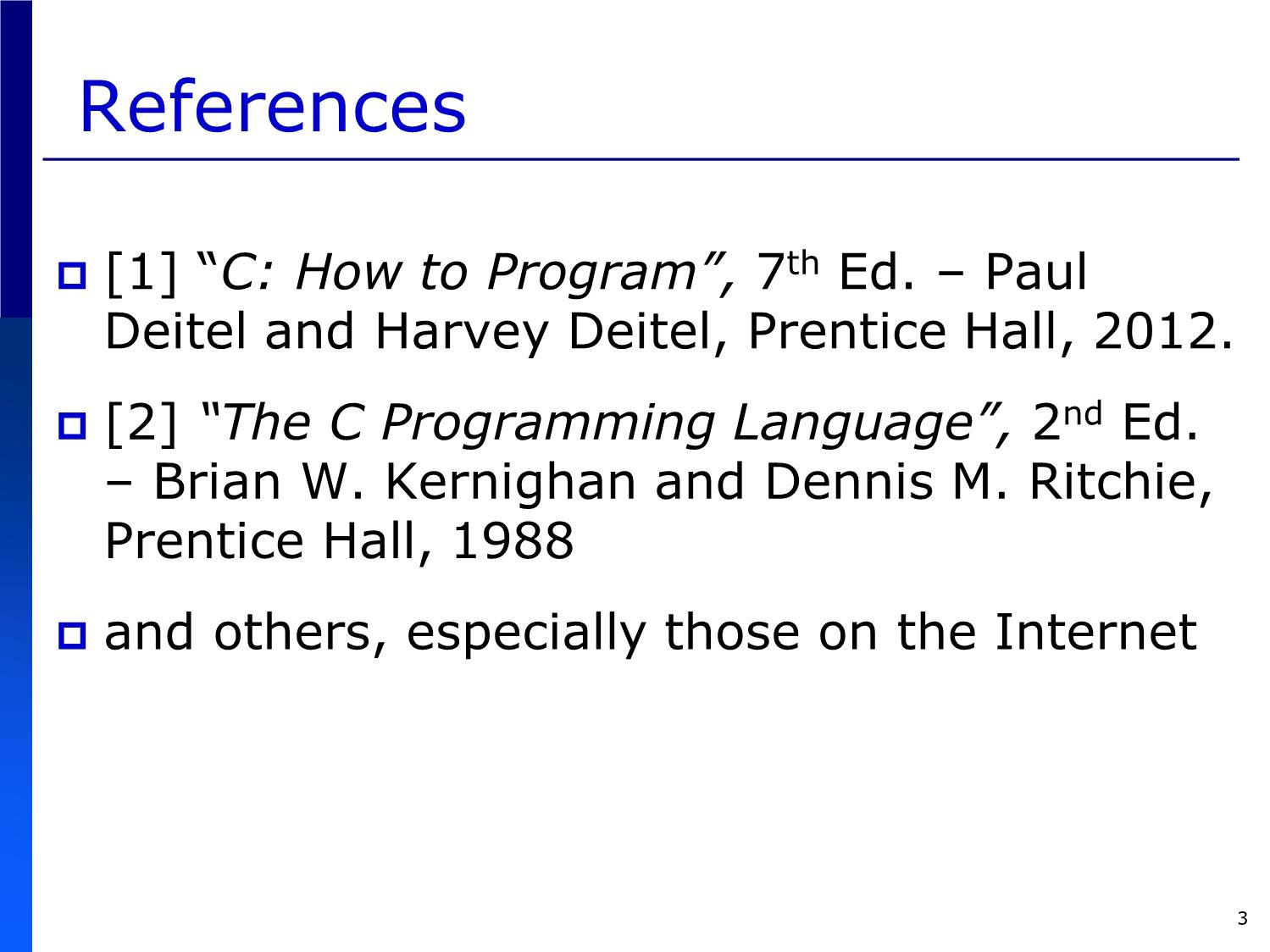
Trang 3
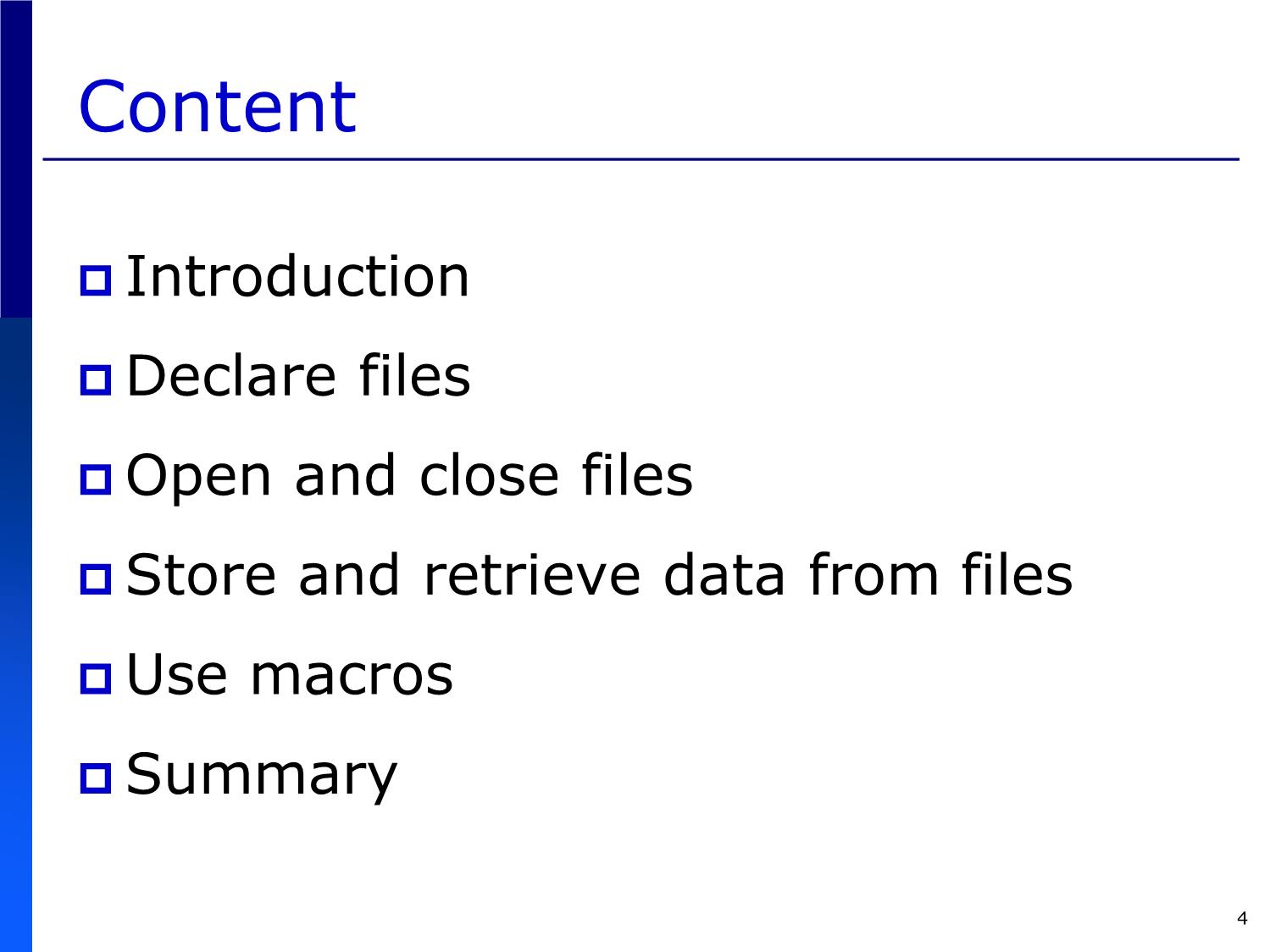
Trang 4
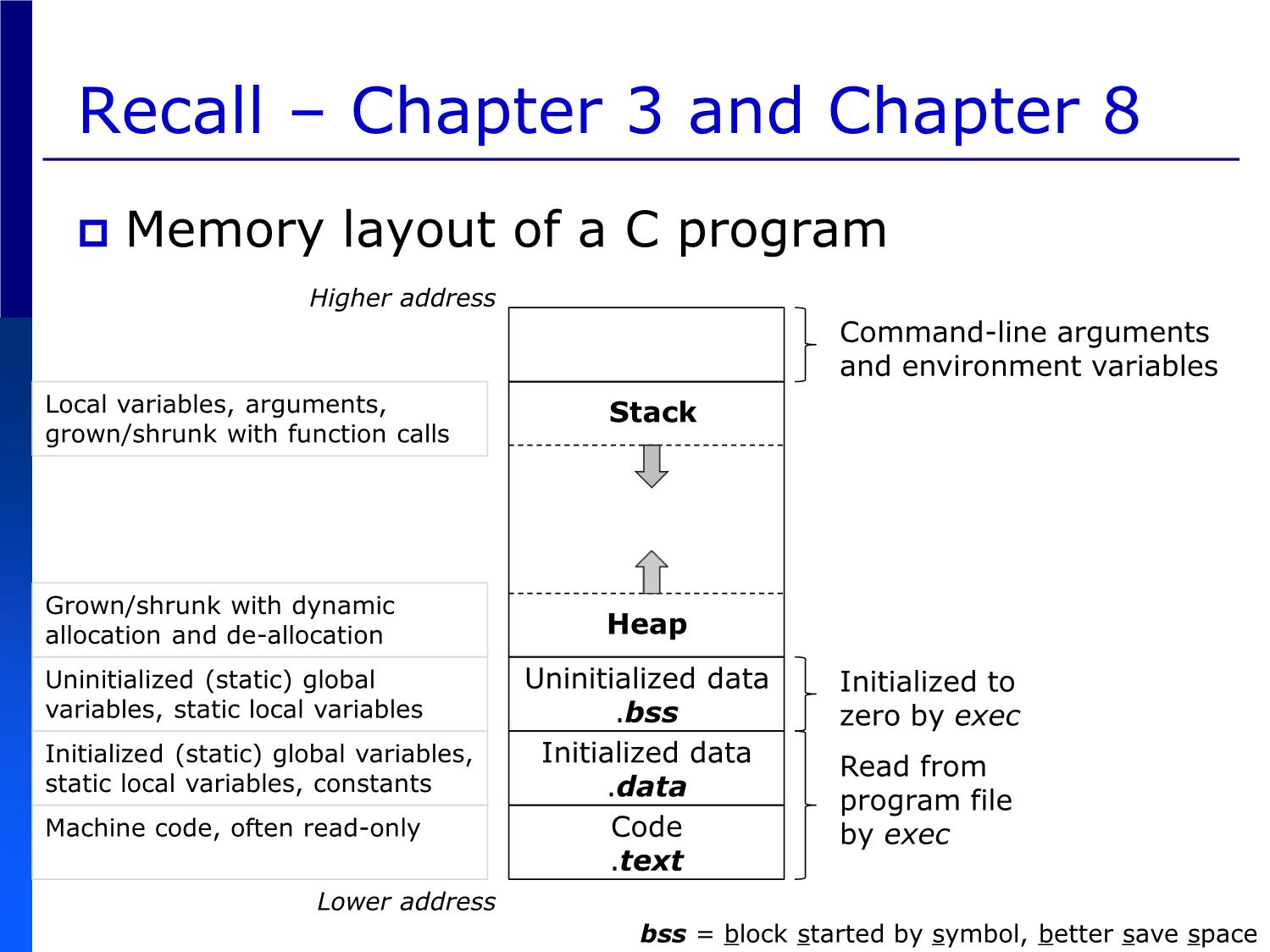
Trang 5
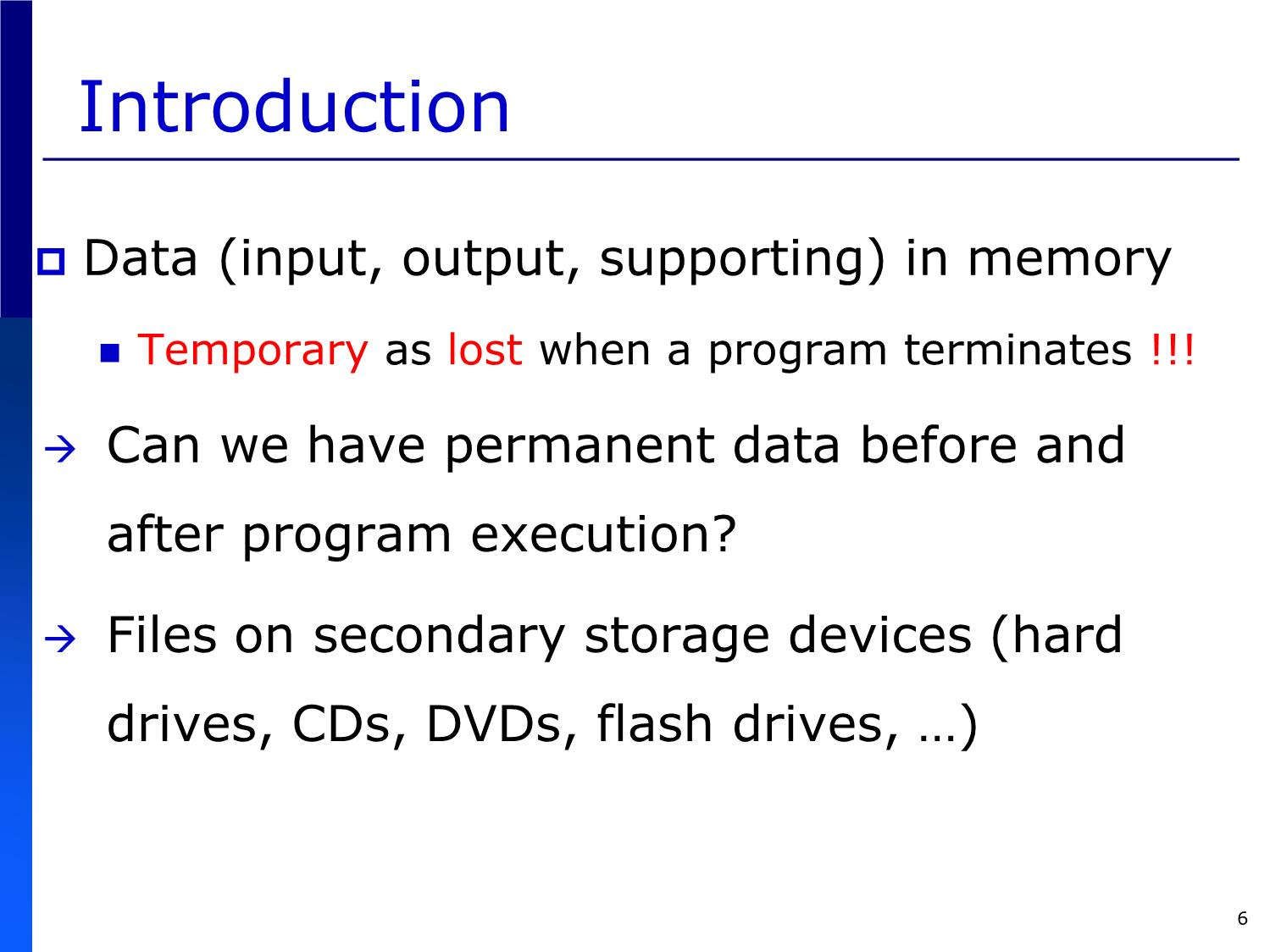
Trang 6
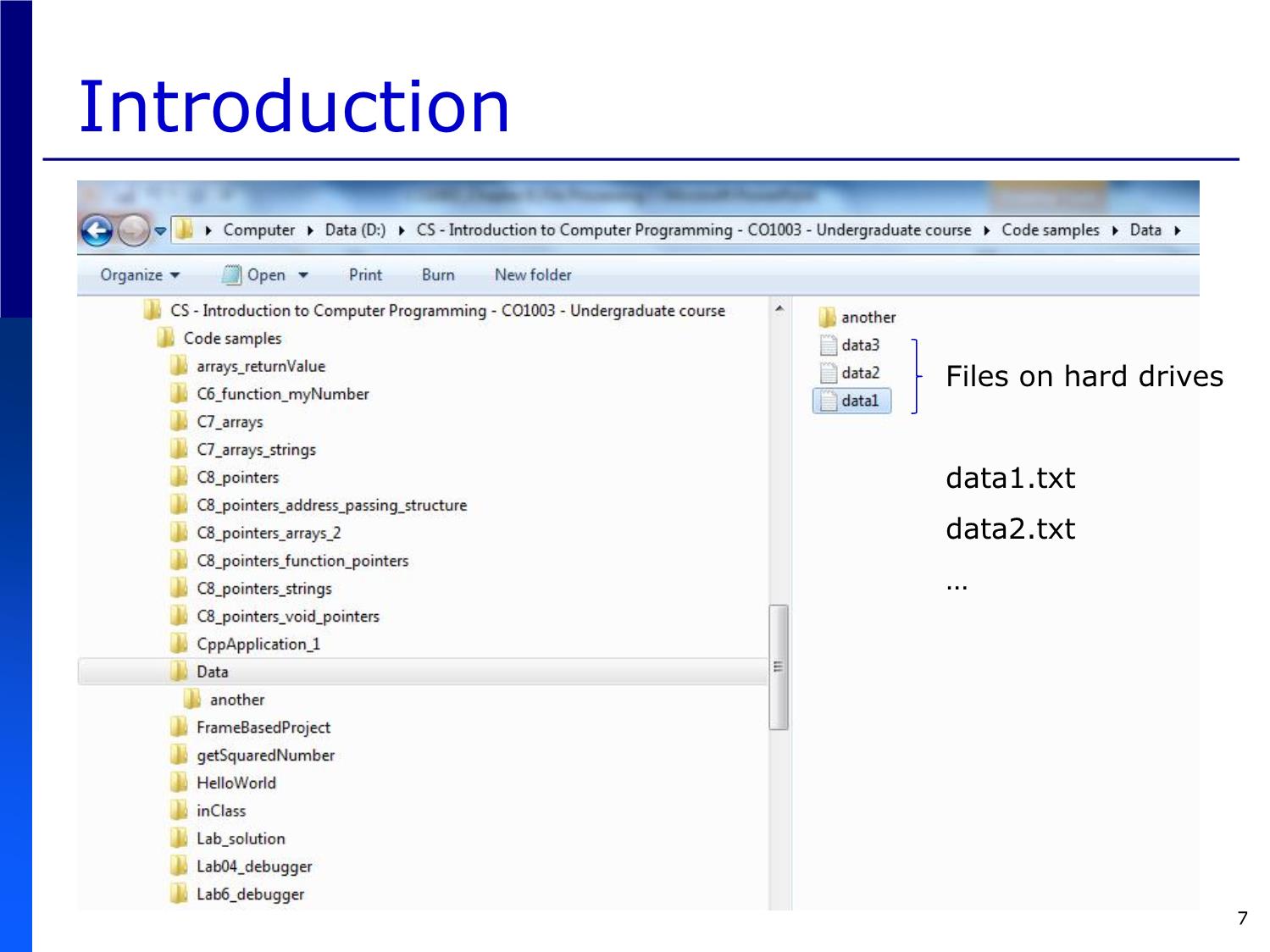
Trang 7
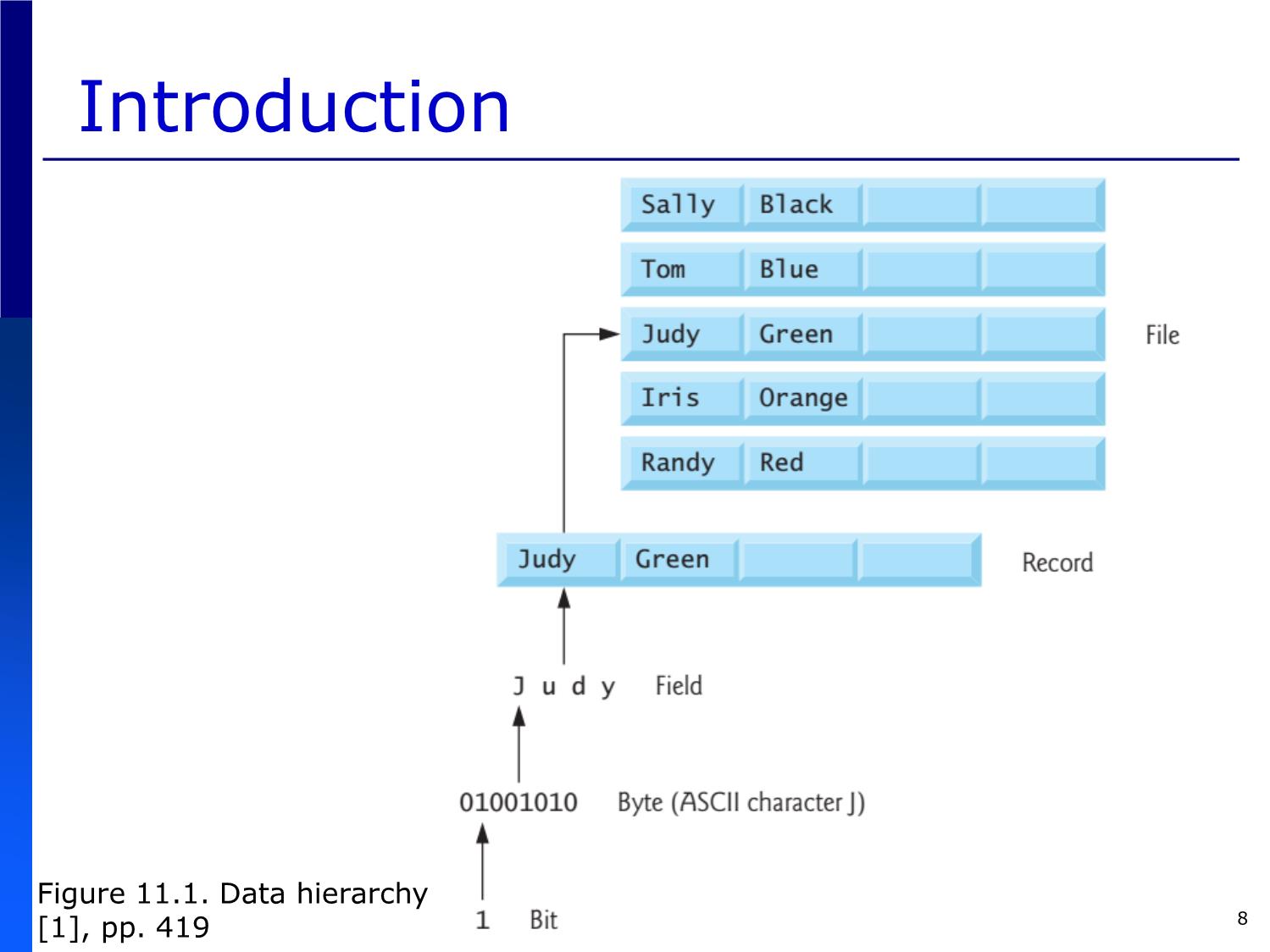
Trang 8
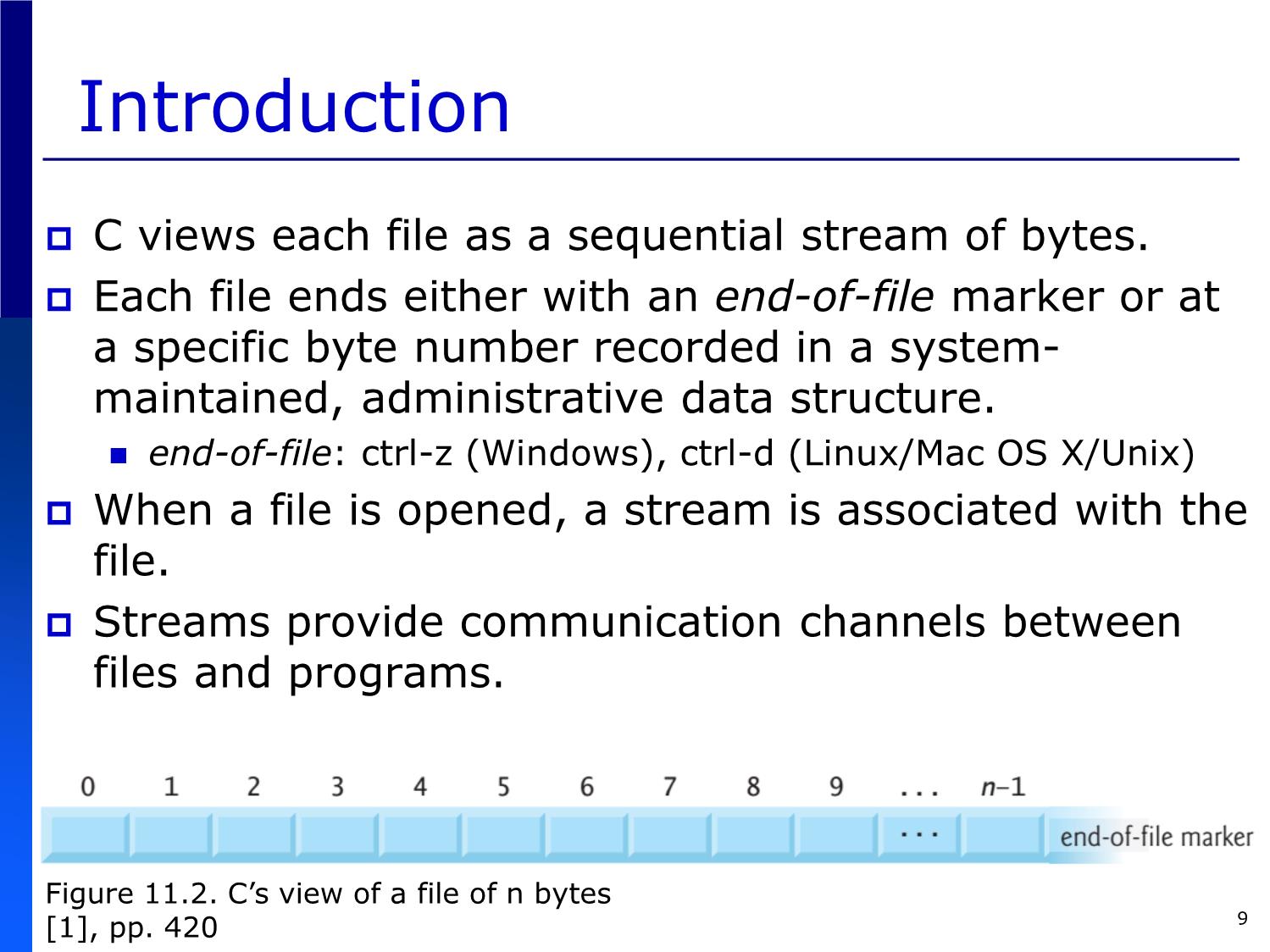
Trang 9
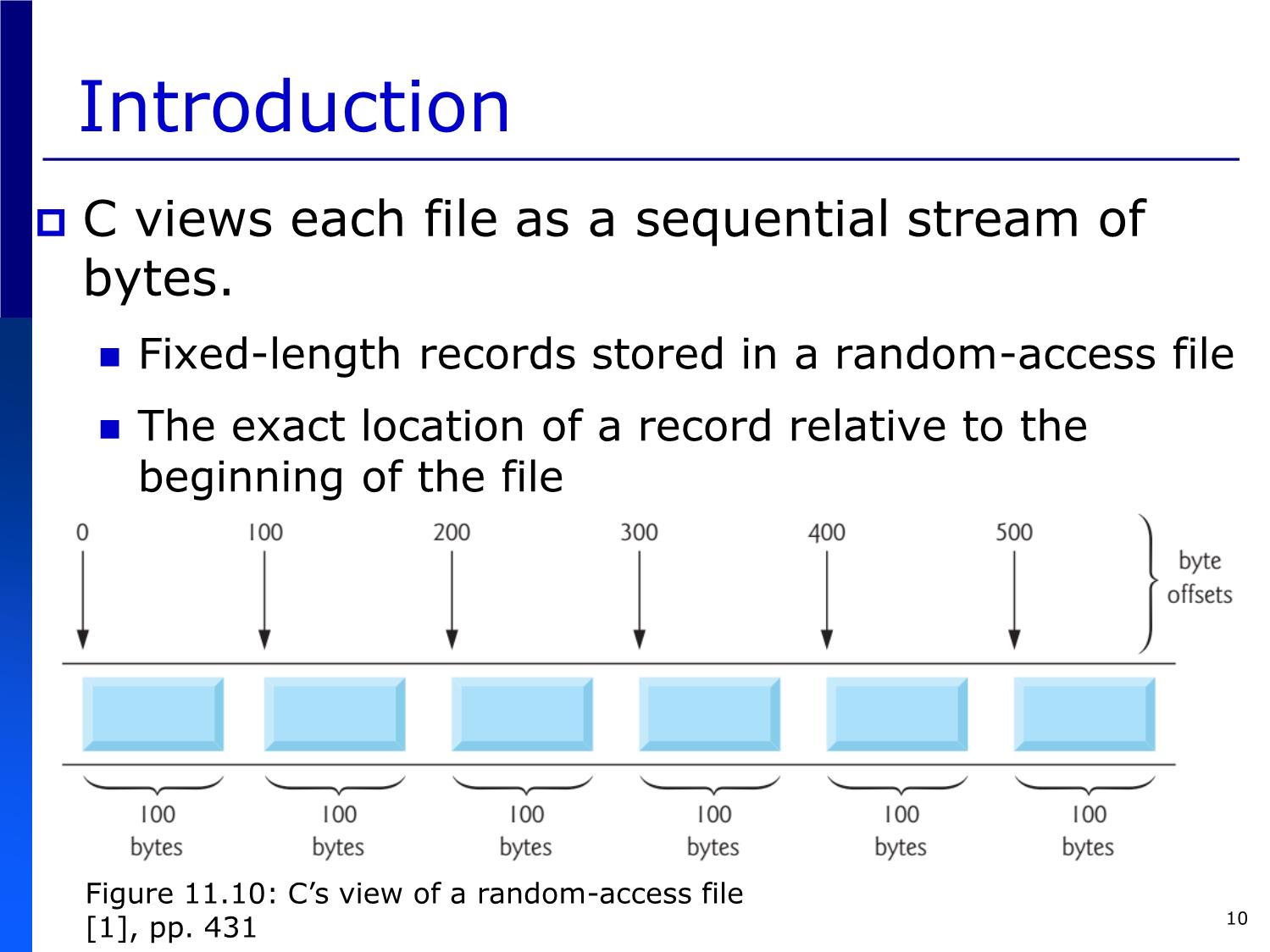
Trang 10
Tải về để xem bản đầy đủ
Bạn đang xem 10 trang mẫu của tài liệu "Bài giảng Introduction to Computer Programming (C language) - Chapter 9: File Processing - Võ Thị Ngọc Châu", để tải tài liệu gốc về máy hãy click vào nút Download ở trên
Tóm tắt nội dung tài liệu: Bài giảng Introduction to Computer Programming (C language) - Chapter 9: File Processing - Võ Thị Ngọc Châu
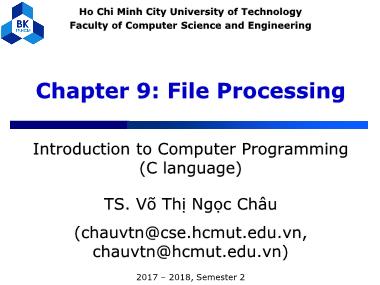
Ho Chi Minh City University of Technology Faculty of Computer Science and Engineering Chapter 9: File Processing Introduction to Computer Programming (C language) TS. Võ Thị Ngọc Châu (chauvtn@cse.hcmut.edu.vn, chauvtn@hcmut.edu.vn) 2017 – 2018, Semester 2 Course Content C.1. Introduction to Computers and Programming C.2. C Program Structure and its Components C.3. Variables and Basic Data Types C.4. Selection Statements C.5. Repetition Statements C.6. Functions C.7. Arrays C.8. Pointers C.9. File Processing 2 References [1] “C: How to Program”, 7th Ed. – Paul Deitel and Harvey Deitel, Prentice Hall, 2012. [2] “The C Programming Language”, 2nd Ed. – Brian W. Kernighan and Dennis M. Ritchie, Prentice Hall, 1988 and others, especially those on the Internet 3 Content Introduction Declare files Open and close files Store and retrieve data from files Use macros Summary 4 Recall – Chapter 3 and Chapter 8 Memory layout of a C program Higher address Command-line arguments and environment variables Local variables, arguments, Stack grown/shrunk with function calls Grown/shrunk with dynamic allocation and de-allocation Heap Uninitialized (static) global Uninitialized data Initialized to variables, static local variables .bss zero by exec Initialized (static) global variables, Initialized data Read from static local variables, constants .data program file Machine code, often read-only Code by exec .text Lower address bss = block started by symbol, better save space Introduction Data (input, output, supporting) in memory Temporary as lost when a program terminates !!! Can we have permanent data before and after program execution? Files on secondary storage devices (hard drives, CDs, DVDs, flash drives, ) 6 Introduction Files on hard drives data1.txt data2.txt 7 Introduction Figure 11.1. Data hierarchy [1], pp. 419 8 Introduction C views each file as a sequential stream of bytes. Each file ends either with an end-of-file marker or at a specific byte number recorded in a system- maintained, administrative data structure. end-of-file: ctrl-z (Windows), ctrl-d (Linux/Mac OS X/Unix) When a file is opened, a stream is associated with the file. Streams provide communication channels between files and programs. Figure 11.2. C’s view of a file of n bytes [1], pp. 420 9 Introduction C views each file as a sequential stream of bytes. Fixed-length records stored in a random-access file The exact location of a record relative to the beginning of the file Figure 11.10: C’s view of a random-access file [1], pp. 431 10 Introduction Operations on files Create Open Read Write (write a new file, append an existing file) Close Access to files in C for processing Sequential access to a sequential stream of bytes Random access to a stream of fixed-size records 11 Introduction The standard library: FILE *fopen(const char *filename, const char *mode) FILE *freopen(const char *filename, const char *mode, FILE *stream) int fclose(FILE *stream) size_t fread(void *ptr, size_t size, size_t nmemb, FILE *stream) int fgetc(FILE *stream) char *fgets(char *str, int n, FILE *stream) int fscanf(FILE *stream, const char *format, ...) size_t fwrite(const void *ptr, size_t size, size_t nmemb, FILE *stream) int fputc(int char, FILE *stream) int fputs(const char *str, FILE *stream) 12 int fprintf(FILE *stream, const char *format, ...) Declare files Each file communicated with C program via a stream controlled by a pointer of FILE identifier: a valid identifier for a pointer FILE* identifier; pointing to a file stream FILE: an object type suitable for storing FILE* pFile1; information for a file stream, defined in the standard library FILE* pFile2 = 0; FILE*: a pointer type of FILE type FILE* pFile3 = NULL; A file pointer will be associated with a file stream once a file is opened. 13 Open and close files Open files FILE *fopen(const char *filename, const char *mode) Opens the file pointed to by filename using the given opening mode. pFile1 = fopen("Data.txt", "r"); Close files int fclose(FILE *stream) Closes the stream. All buffers are flushed. fclose(pFile1); 14 Open and close files Open files Filename = "Data.txt" Located in the current directory Filename = ".\\Data\\Data.txt" Located in the sub-directory of the current directory Filename = "..\\Data.txt" Located in the super-directory of the current directory Filename = "D:\\CS - Introduction to Computer Programming - CO1003 - Undergraduate course\\Code samples\\Data\\Data.txt" Located in the specified directory with the absolute path 15 Open and close files File opening modes 16 Store and retrieve data from files Store data into files (write) A sequence of bytes from memory to the file The file plays a role of stdout. Retrieve data from files (read) A sequence of bytes from the files to memory The file plays a role of stdin. What bytes? Read (file -> memory) Write (memory -> file) A char fgetc fputc A line of bytes fgets fputs Formatted bytes fscanf fprintf Bytes in the fread fwrite binary mode 17 Store and retrieve data from files 18 Store and retrieve data from files 19 Store and retrieve data from files What bytes? Read (file -> memory) Write (memory -> file) A char fgetc fputc char aChar; aChar = fgetc(pFile1); fputc('a', pFile1); A line of bytes fgets fputs char aStr[50]; fgets(aStr, 50, pFile1); fputs("Today?", pFile1); Formatted bytes fscanf fprintf int anInt; fscanf(pFile1, "%d %c fprintf(pFile1, "%d %d char aChar; %f", &anInt, &aChar, %c %f", 10, anInt, aChar, float aFloat; &aFloat); aFloat); Bytes in the fread fwrite binary mode char buffer[50]; fread(buffer, fwrite(buffer, sizeof(int)+sizeof(char)+si strlen(buffer)+1, 1, zeof(float)+1, 5, pFile1); pFile1); 20 Process the grades of each student Put them all togetherin a file for their averaged grades. 21 Process the grades of each student in a file for their averaged grades. Put them all togetherWrite the resulting averaged grades into another file. 22 Input data file Put them all together Output data file in the binary mode Output data file in the formatted mode 23 Output data file in the binary mode Output data file in the formatted mode 24 Use macros Macro An identifier in #define preprocessor directive #define identifier replacement-text identifier: a valid identifier scoped from its definition to the end of the file or to the #undef directive replacement-text: text on the line or longer text on many lines with a backslash (\) Considered as operations defined as symbols With no argument processed like a symbolic constant With arguments processed like an inline function . Arguments are substituted in the replacement text . The replacement text then replaces the identifier and argument list in the program 25 Use macros #define identifier replacement-text User-defined macros Parentheses need using for correct value determination. 26 Use macros #define identifier replacement-text #define CIRCUMFERENCE(radius) (2*PI*(radius)) r=1.5 CIRCUMFERENCE(r+1) = (2*PI*(r+1)) = 15.71 VS. #define CIRCUMFERENCE(radius) (2*PI*radius) r=1.5 CIRCUMFERENCE(r+1) = (2*PI*r+1) = 10.42 X 27 Use macros #define identifier replacement-text #define DIAMETER(radius) ((radius) + (radius)) CIRCUMFERENCE = PI*DIAMETER(r+1) r=1.5 = PI*((r+1)+(r+1)) = 15.71 VS. #define DIAMETER(radius) (radius) + (radius) CIRCUMFERENCE = PI*DIAMETER(r+1) r=1.5 = PI*(r+1)+(r+1) = 10.35 X 28 Use macros Conditional compilation up to macro definition control the execution of preprocessor directives and the compilation of program code #ifdef identifier //if identifier is defined, consider the following code #endif #ifdef identifier //if identifier is defined, consider the following code #else //else consider the following code #endif #ifdef identifier //if identifier is defined, consider the following code #elif constant-expression #endif #ifndef identifier //if identifier is not defined, consider the following code #endif #undef identifier //remove the definition of identifier 29 Use macros – Conditional compilation Recall – Chapter 6 – Functions Header file: mynumber.h 30 Use macros Many macros predefined in the standard library files FLT_MIN: the minimum finite floating-point value FLT_MAX: the maximum finite floating-point value INT_MIN: the minimum value for an int with 2 bytes INT_MAX: the maximum value for an int with 2 bytes LC_ALL: sets everything with the location specific settings LC_CTYPE: affects all the character functions with settings 31 Use macros Many macros predefined in the standard library files The stdarg.h header defines a variable type va_list and three following macros which can be used to get the arguments in a function when the number of arguments are not known i.e. variable number of arguments. . void va_start(va_list ap, last_arg) . type va_arg(va_list ap, type) . void va_end(va_list ap) A function of variable arguments is defined with the ellipsis (,...) at the end of the parameter list. 32 Use macros - va_list A type whose variable ap is used for holding information about the arguments void va_start(va_list ap, last_arg) The macro initializes ap variable to be used with the va_arg and va_end macros. The last_arg is the last known fixed argument being passed to the function i.e. the argument before the ellipsis. type va_arg(va_list ap, type) The macro retrieves the next argument in the parameter list of the function with type type. void va_end(va_list ap) The macro allows a function with variable arguments which used the va_start macro to return. If va_end is not called before returning from the function, the result is undefined. 33 Use macros - Define the function isSubstring to check if a substring is contained by a list of given strings. 34 Use macros - Variable ap is required to have an access to each argument in the argument list after this point. This macro is required to start the access to the argument list. This macro is required to have an access to an actual argument of type char* in the argument list. This macro is required to end the access to the argument list before the return of the function. 35 Summary File processing in C Allows data permanency Performs with the standard functions in the library Open/Close Read/Write/Append Supports two access schemes Sequential access Random access 36 Summary Macros in the #define preprocessor directive User-defined macros vs. Macros in the libraries Conditional compilation based on macro definitions Examples with macros in the for functions with the variable numbers of arguments 37 Chapter 9: File Processing 38
File đính kèm:
bai_giang_introduction_to_computer_programming_c_language_ch.pdf